A built-in data structure in Python called a dictionary lets you store and retrieve data using key-value pairs. To put it another way, a Python dictionary is a group of items, each of which is a pair made up of a key and a value. The value that corresponds to the key can be accessed using the key as a distinctive identifier.
Looking to Learn Python? Book a Free Trial Lesson and match with top Python Tutors for concepts, projects and assignment help on Wiingy today!
What is a Python Dictionary?
Python dictionaries are implemented as hash tables, making them ideal for quick access and modification. For a variety of applications, including web development, data science, and machine learning, they are a very helpful data structure.
Purpose and Benefits of Dictionaries
The main objective of dictionaries is to offer a quick and effective method of organizing and accessing data. Because of this, they are especially helpful in applications where you need to look up data using a special key or identifier. For example, a dictionary might be used to store information about a group of people, where each person’s name is the key and their age is the value.
Some of the benefits of using dictionaries include:
- Fast access and modification of data
- Efficient storage of large amounts of data
- Easy to read and understand code
- Flexible data structure that can be customized to suit your needs
Comparison to Other Data Structures
One of the many data structures available in Python is dictionaries. Besides these, lists, tuples, and sets are some other typical data structures. The best data structure to use will depend on the particular needs of your application and has its own advantages and disadvantages.
Fast access to data based on a key is one of dictionaries’ main advantages over other data structures. On the other hand, lists and tuples require you to loop through the entire collection in order to find a particular item. Due to the fact that sets don’t store values as key-value pairs, they are also less effective than dictionaries for this kind of operation.
Creating a Python Dictionary
Here are the ways of creating python dictionary:
Basic Syntax
The basic syntax for creating a dictionary in Python is as follows:
1my_dict = { key1: value1, key2: value2, key3: value3 }
In this example, my_dict is the name of the dictionary, and key1, key2, and key3 are the keys that are associated with their corresponding values, value1, value2, and value3. Note that the keys and values can be of any data type, and can even be different data types within the same dictionary.
Creating an Empty Python Dictionary
To create an empty dictionary, you can use the following syntax:
1my_dict = {}
In this case, my_dict is simply an empty dictionary with no key-value pairs.
Creating a Dictionary with Initial Values
You can also create a dictionary with initial values using the following syntax:
1my_dict = dict(key1=value1, key2=value2, key3=value3)
This syntax is similar to the basic syntax, but uses the dict() function instead of the curly braces. The result is the same as in the previous example, where my_dict is a dictionary with the specified key-value pairs.
Accessing and Modifying Dictionary Elements
Accessing Dictionary Elements
To access an element in a dictionary, you can use the key as follows:
1my_dict = { "name": "Alice", "age": 25, "city": "New York" } name = my_dict["name"] age = my_dict["age"] city = my_dict["city"]
In this example, my_dict is a dictionary with three key-value pairs. To access the value associated with the key “name”, we use my_dict[“name”], which returns the value “Alice”. Similarly, to access the value associated with the key “age”, we use my_dict[“age”], which returns the value 25. Finally, to access the value associated with the key “city”, we use my_dict[“city”], which returns the value “New York”.
Note that if you try to access a key that does not exist in the dictionary, you will get a KeyError:
1my_dict = { "name": "Alice", "age": 25, "city": "New York" } # Trying to access a key that does not exist # will result in a KeyError country = my_dict["country"]
Modifying Dictionary Elements
To modify the value associated with a key in a dictionary, you can simply assign a new value to that key:
1my_dict = { "name": "Alice", "age": 25, "city": "New York" }
2# Update the value associated with the "age" key
3my_dict["age"] = 26
4# The dictionary now contains the updated value
5print(my_dict)
6# Output: {"name": "Alice", "age": 26, "city": "New York"}
In this example, we modify the value associated with the “age” key by assigning a new value of 26. The resulting dictionary now has the updated value for the “age” key.
Adding New Elements to a Dictionary
To add a new key-value pair to a dictionary, you can simply assign a value to a new key:
1my_dict = { "name": "Alice", "age": 25, "city": "New York" } # Add a new key-value pair my_dict["country"] = "USA" # The dictionary now contains the new key-value pair print(my_dict) # Output: {"name": "Alice", "age": 25, "city": "New York", "country": "USA"}
In this example, we add a new key-value pair “country”: “USA” to the dictionary by assigning a value to a new key “country”. The resulting dictionary now contains the new key-value pair.
Removing Elements from a Dictionary
To remove a key-value pair from a dictionary, you can use the del keyword:
1my_dict = { "name": "Alice", "age": 25, "city": "New York" } # Remove the key-value pair associated with the "city" key del my_dict["city"] # The dictionary no longer contains the removed key-value pair print(my_dict) # Output: {"name": "Alice", "age": 25}
In this example, we remove the key-value pair associated with the “city” key using the del keyword. The resulting dictionary no longer contains the removed key-value pair.
Dictionary Methods
Common Dictionary Methods
In addition to accessing, modifying, and adding elements to a dictionary, there are a number of other methods that can be used to manipulate dictionaries. Some of the most common dictionary methods include:
- clear(): Removes all key-value pairs from the dictionary.
- copy(): Returns a shallow copy of the dictionary.
- get(key, default=None): Returns the value associated with the specified key, or the default value if the key is not in the dictionary.
- items(): Returns a view object that contains the key-value pairs of the dictionary as tuples.
- keys(): Returns a view object that contains the keys of the dictionary.
- pop(key, default=None): Removes and returns the value associated with the specified key, or the default value if the key is not in the dictionary.
- popitem(): Removes and returns the last inserted key-value pair from the dictionary as a tuple.
- values(): Returns a view object that contains the values of the dictionary.
Using the keys(), values(), and items() Methods
The keys(), values(), and items() methods can be used to access the keys, values, and key-value pairs of a dictionary, respectively. These methods return view objects that can be used to iterate over the corresponding elements of the dictionary. For example:
1my_dict = { "name": "Alice", "age": 25, "city": "New York" } # Using the keys() method for key in my_dict.keys(): print(key)
2# Output: # "name" # "age" # "city"
3# Using the values() method for value in my_dict.values(): print(value)
4# Output: # "Alice" # 25 # "New York"
5# Using the items() method for key, value in my_dict.items(): print(key, value)
6# Output: # "name" "Alice" # "age" 25 # "city" "New York"
Merging Dictionaries with update()
The update() method can be used to merge the key-value pairs of one dictionary into another. This method takes a dictionary as an argument and updates the calling dictionary with the key-value pairs from the argument dictionary. For example:
1my_dict = { "name": "Alice", "age": 25, "city": "New York" } new_dict = { "country": "USA", "state": "NY" } # Merge the new_dict into the my_dict my_dict.update(new_dict) # The my_dict now contains the merged key-value pairs print(my_dict)
2 # Output: {"name": "Alice", "age": 25, "city": "New York", "country": "USA", "state": "NY"}
In this example, we merge the new_dict into the my_dict using the update() method. The resulting my_dict now contains the merged key-value pairs.
Sorting Dictionaries with sorted()
Dictionaries in Python are unordered, which means that they do not guarantee that the elements will be in a specific order. However, it is possible to sort the key-value pairs of a dictionary based on the keys using the sorted() function. The sorted() function takes an iterable object as an argument and returns a new sorted list. For dictionaries, the sorted() function can be used with the items() method to sort the key-value pairs based on the keys. For example:
1my_dict = { "name": "Alice", "age": 25
Best Practices
Dictionaries in Python are flexible data structures that can be applied in a variety of contexts. However, there are some best practices that you should keep in mind when working with dictionaries:
Choosing Appropriate Keys
Keys ought to be distinct and unchangeable. Additionally, selecting descriptive and simple to understand keys is crucial.
Understanding Dictionary Performance
When it comes to accessing and changing values using keys, dictionaries are very quick. However, adding or deleting values might take a while.
Handling Missing Keys
You will receive a KeyError when attempting to access a key that isn’t listed in a dictionary. Use the get() method, which returns a default value if the key doesn’t exist, to prevent this from happening.
Code Styling
It’s crucial to adhere to best practices for code styling when using Python, including giving your variables descriptive names, adding comments, and using whitespace to make your code easier to read.
Built-in Dictionary Methods
There are several built-in methods that are available to you for manipulating dictionaries in Python. Some of the most popular techniques are listed below:
A. d.clear(): This method removes all the items from the dictionary.
B. d.get(<key>[, <default>]): This method returns the value for a given key. If the key is not found, it returns a default value (which defaults to None).
C. d.items(): This method returns a list of key-value pairs in the dictionary.
D. d.keys(): This method returns a list of keys in the dictionary.
E. d.values(): This method returns a list of values in the dictionary.
F. d.pop(<key>[, <default>]): This method removes and returns the value for a given key. If the key is not found, it returns a default value (which defaults to None).
G. d.popitem(): This method removes and returns an arbitrary key-value pair from the dictionary.
H. d.update(<obj>): This method updates the dictionary with key-value pairs from another dictionary or iterable object.
Conclusion
A versatile data structure that can be used in many different applications is the dictionary in Python. They come with a set of built-in methods that make them simple to use and offer quick and effective access to values using keys.
We discussed the fundamentals of working with dictionaries in this article, including how to create and access them, edit and add elements, and use dictionary methods. We also discussed some built-in techniques and best practices that you can use to enhance your code.
Overall, learning how to use dictionaries will make you a more effective and efficient developer because they are a fundamental component of Python programming.
Any programmer should have Python dictionaries in their toolbox. By understanding the basics of how they work and the different ways they can be used, you will be able to write more efficient and effective code.
Looking to Learn Python? Book a Free Trial Lesson and match with top Python Tutors for concepts, projects and assignment help on Wiingy today!
FAQs:
What is a Python dictionary?
A Python dictionary is an unordered collection of key-value pairs. Each key is unique and maps to a corresponding value. Dictionaries are mutable, meaning their contents can be modified after they are created. They are often used to store and retrieve data in a way that allows for efficient lookups based on keys.
How to create a Python dictionary?
To create a Python dictionary, you can use curly braces {} and separate each key-value pair with a colon (:), like this:
my_dict = {‘key1’: ‘value1’, ‘key2’: ‘value2’, ‘key3’: ‘value3’}
Alternatively, you can use the built-in dict() constructor function and pass in key-value pairs as arguments, like this:
my_dict = dict(key1=’value1′, key2=’value2′, key3=’value3′)
What is __dict__ in Python dictionary?
In Python, __dict__ is a special attribute of an object that returns a dictionary containing the object’s attributes. For example, you can use __dict__ to inspect the attributes of a class or instance:
class MyClass: def __init__(self, name): self.name = name my_obj = MyClass(“John”) print(my_obj.__dict__)
Output:
{‘name’: ‘John’}
What is the difference between dict() and {} in Python?
Both dict() and {} can be used to create dictionaries in Python. However, there is a subtle difference between the two.
{} is a literal syntax for creating an empty dictionary, while dict() is a built-in constructor function that can create a dictionary with optional initial values.
For example, to create an empty dictionary, you can use either {} or dict():
my_dict = {} my_dict = dict()
To create a dictionary with initial values, you can use dict() and pass in key-value pairs as arguments:
my_dict = dict(key1=’value1′, key2=’value2′)
On the other hand, using {} to create a dictionary with initial values requires you to separate each key-value pair with a comma:
my_dict = {‘key1’: ‘value1’, ‘key2’: ‘value2’}
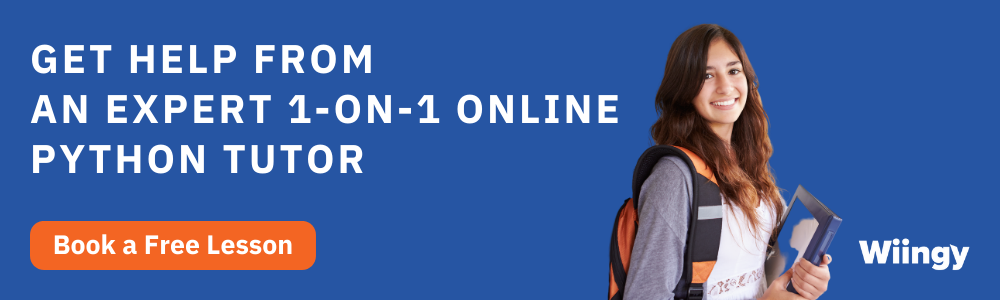
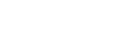
Jan 30, 2025
Was this helpful?