Python is a well-liked programming language that can be used for many things, including web development, scientific computing, and data analysis. Python numbers are a fundamental data type in programming and are used to perform arithmetic operations.
Importance of Python Numbers in Programming:
Programming frequently involves numbers, and Python provides a number of numerical data types. These numerical data types are essential for a number of programming tasks, including data analysis, statistical modeling, and mathematical operations. Therefore, any student hoping to learn programming must understand Python numbers.
Looking to Learn Python? Book a Free Trial Lesson and match with top Python Tutors for concepts, projects and assignment help on Wiingy today!
Number Types in Python
Here are the number types in python:
A. Integers:
Whole numbers are represented as integers in Python by the int() data type. For example, 3, 42, and -15 are all integers. Python supports arithmetic operations such as addition, subtraction, multiplication, and division on integers.
Example:
1a = 10 b = 5 c = a + b print(c) # Output: 15
B. Floating-Point Numbers:
Numbers with a decimal point, also referred to as floating-point numbers or floats, are represented by the float() data type in Python. For example, 2.5, 3.1416, and -0.75 are all floats. Python supports arithmetic operations such as addition, subtraction, multiplication, and division on floats.
Example:
1a = 2.5 b = 1.5 c = a * b print(c) # Output: 3.75
C. Complex Numbers:
Python’s complex() data type represents complex numbers. A complex number consists of a real and imaginary part. For example, 3 + 4j and -2 – 6j are complex numbers. Python supports arithmetic operations such as addition, subtraction, multiplication, and division on complex numbers.
Example:
1a = 3 + 4j b = 1 - 2j c = a * b print(c) # Output: (11+2j)
III. Basic Operations on Numbers
A. Arithmetic Operators and Expressions:
Python supports arithmetic operators such as +, -, *, and / for performing arithmetic operations on numbers. For example:
1a = 10 b = 5 c = a + b print(c) # Output: 15
Expressions can be used to combine multiple arithmetic operations. For example:
1a = 10 b = 5 c = 3 d = (a + b) * c print(d) # Output: 45
B. Modulus and Floor Division:
Python supports the modulus operator (%) for finding the remainder of a division operation. For example:
1a = 10 b = 3 c = a % b print(c) # Output: 1
Python also supports the floor division operator (//), which returns the quotient of a division operation rounded down to the nearest integer. For example:
1a = 10 b = 3 c = a // b print(c) # Output: 3
C. Exponentiation:
Python supports the exponentiation operator (**) for calculating exponents. For example:
1a = 2 b = 3 c = a ** b print(c) # Output: 8
D. Comparison Operators:
Python supports comparison operators such as ==, !=, <, >, <=, and >= for comparing numbers. These operators return a Boolean value (True or False) depending on whether the comparison is true or false. For example:
1a = 10 b = 5 c = a > b print(c) # Output: True
In the above example, the comparison operator ‘>’ compares the value of ‘a’ and ‘b’. As ‘a’ is greater than ‘b’, the output is True.
Numeric Functions and Constants
A. abs(), pow(), round():
The built-in Python numeric functions abs(), pow(), and round() are just a few examples.
- The abs() function returns the absolute value of a number.
- The pow() function returns the result of raising a number to a specified power.
- The round() function rounds a number to a specified number of decimal places.
Example:
1a = -5 b = 3 c = abs(a) # Output: 5 d = pow(b, 2) # Output: 9 e = round(3.14159, 2) # Output: 3.14
B. min() and max():
The built-in Python functions min() and max() are also available to determine the minimum and maximum values in a sequence, respectively.
Example:
1a = [1, 2, 3, 4, 5] b = min(a) # Output: 1 c = max(a) # Output: 5
C. math module:
Python’s math module provides access to a range of mathematical functions, constants, and expressions. Some of the commonly used functions include:
- Trigonometric functions: sin(), cos(), tan(), asin(), acos(), and atan().
- Logarithmic functions: log(), log10(), log2(), and exp().
- Constants: pi, e, and tau.
Example:
1import math a = math.sin(math.pi / 2) # Output: 1.0 b = math.log(2) # Output: 0.6931471805599453 c = math.pi # Output: 3.141592653589793
Converting Between Number Types
A. Typecasting:
Python supports typecasting, the transformation of one data type into another. This is especially useful when dealing with different number types. For example:
1a = 10 b = 2.5 c = float(a) # Output: 10.0 d = int(b) # Output: 2
B. Hexadecimal, Octal, and Binary Representations:
Python supports hexadecimal, octal, and binary representations of numbers. These can be useful when working with low-level programming, such as computer hardware or networking.
Example:
1a = 0xFF # hexadecimal representation of 255 b = 0o10 # octal representation of 8 c = 0b1010 # binary representation of 10
Numeric Sequences
A. Range() function:
Python’s range() function is used to generate a sequence of numbers. It takes three arguments: start, stop, and step.
Example:
1a = range(1, 10, 2) # Output: [1, 3, 5, 7, 9]
B. List and Tuple Sequences:
Python allows creating lists and tuples that can hold a sequence of numbers.
Example:
1a = [1, 2, 3, 4, 5] # List b = (1, 2, 3, 4, 5) # Tuple
C. Using Loops with Sequences:
Loops can be used to iterate over a sequence of numbers and perform operations on them.
Example:
1a = [1, 2, 3, 4, 5] for i in a: print(i * 2) # Output: 2, 4, 6, 8, 10
Random Numbers
A. random module:
The random module in Python offers tools for creating random numbers. Applications like games, simulations, and cryptography can all benefit from this.
Example:
1import random a = random.random() # Output: a random float between 0 and 1 b = random.randint(1, 10) # Output: a random integer between 1 and 10
B. Generating random integers and floating-point numbers:
Numerous functions are available in the random module of Python to produce random floating-point and integer numbers. The functions include:
- randrange(): generates a random integer between start and stop values with an optional step value.
- uniform(): generates a random floating-point number between start and stop values.
Example:
1import random a = random.randrange(1, 10, 2) # Output: a random odd integer between 1 and 9 b = random.uniform(0, 1) # Output: a random float between 0 and 1
C. Random choices and shuffling:
The random module in Python also has functions for randomly selecting items from a sequence and shuffling a sequence.
Example:
1import random a = random.choice([1, 2, 3, 4, 5]) # Output: a random choice from the list b = random.shuffle([1, 2, 3, 4, 5]) # Output: the list shuffled randomly
Bitwise Operations on Numbers
A. Bitwise AND, OR, XOR, and NOT:
Python supports bitwise operations on integers. The bitwise operations include:
- Bitwise AND (&): returns 1 if both bits are 1.
- Bitwise OR (|): returns 1 if at least one bit is 1.
- Bitwise XOR (^): returns 1 if only one bit is 1.
- Bitwise NOT (~): inverts all the bits in a number.
Example:
1a = 0b1010 # binary representation of 10 b = 0b1100 # binary representation of 12 c = a & b # Output: binary representation of 8 (0b1000) d = a | b # Output: binary representation of 14 (0b1110) e = a ^ b # Output: binary representation of 6 (0b0110) f = ~a # Output: binary representation of -11 (0b11111111111111111111111111110101)
B. Bit Shifts:
Python also supports bit shifts, which move the bits in a number left or right.
Example:
1a = 0b0010 # binary representation of 2 b = a << 1 # Output: binary representation of 4 (0b0100) c = a >> 1 # Output: binary representation of 1 (0b0001)
Complex Numbers
A. Creating Complex Numbers:
Complex numbers, which have a real part and an imaginary part, are supported in Python. Complex numbers can be created using the complex() function.
Example:
1a = complex(1, 2) # Output: 1+2j
B. Arithmetic Operations on Complex Numbers:
Python supports arithmetic operations on complex numbers. The arithmetic operations include:
- Addition (+)
- Subtraction (-)
- Multiplication (*)
- Division (/)
Example:
1a = complex(1, 2) b = complex(3, 4) c = a + b # Output: 4+6j d = a * b # Output: -5+10j
C. Complex Number Functions:
Python also supports several functions that can be used with complex numbers. Some of these functions include:
- abs(): returns the magnitude (or absolute value) of a complex number.
- conjugate(): returns the conjugate of a complex number.
- real(): returns the real part of a complex number.
- imag(): returns the imaginary part of a complex number.
Example:
1a = complex(1, 2) b = abs(a) # Output: 2.23606797749979 c = a.conjugate() # Output: 1-2j d = a.real # Output: 1.0 e = a.imag # Output: 2.0
These are some of the basic concepts and operations related to Python numbers that are important for Class 6 US students to learn. It is recommended to practice these concepts through examples and exercises to gain proficiency.
Conclusion
The fundamentals of Python numbers have been covered in this guide, including the number types (integers, floating-point numbers, and complex numbers), fundamental operations (arithmetic, modulus, floor division, exponentiation, and comparison), numerical functions and constants, converting between number types, numeric sequences, random numbers, bitwise operations, and complex numbers.
Practical applications of Python Numbers:
Python numbers are crucial for programming because they are used in a wide range of tasks, such as data analysis, scientific computing, and web development. Python numbers can be used to solve mathematical problems, create graphs and charts, and analyze huge datasets, among other practical uses.
Looking to Learn Python? Book a Free Trial Lesson and match with top Python Tutors for concepts, projects and assignment help on Wiingy today!
FAQs
What is Python numbers?
Python numbers are used to represent numerical data in Python programming language. They include integers, floating-point numbers, and complex numbers.
What are the 3 types of numbers in Python?
The 3 types of numbers in Python are integers, floating-point numbers, and complex numbers.
How do you use numbers in Python?
You can use numbers in Python by assigning them to variables and using them in arithmetic operations, comparisons, and other operations.
How do you get numbers from 1 to 100 in Python?
You can use the range() function to generate numbers from 1 to 100 in Python, like this:
for i in range(1, 101): print(i)
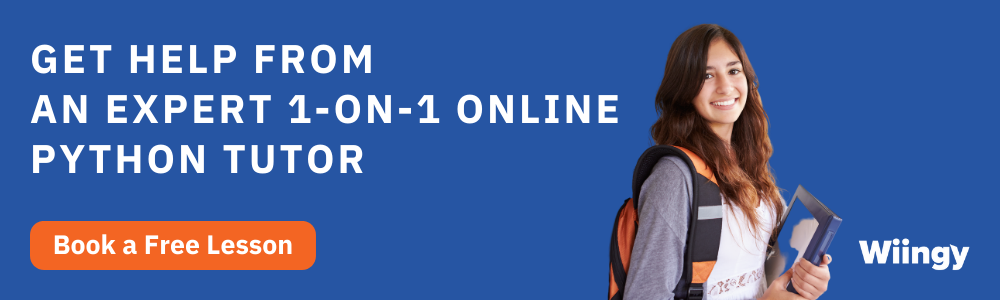
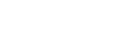
Jan 30, 2025
Was this helpful?