What is File Handling in Python?
Python is a popular programming language that can be used for a wide range of tasks, such as making web apps and analyzing data. Every computer language needs to handle input and output, and Python is no different. Your Python programs will be more dependable and user-friendly if you handle input and output [(I/O) handing] properly. This guarantees that they can communicate with the user and other systems in an effective manner.
This article will give a full explanation of how Python handles input and output. It will cover topics like basic input and output, reading and writing files, formatting output, and handling errors. You will have a firm understanding of how to handle input and output in your Python applications by the end of this article.
Looking to Learn Python? Book a Free Trial Lesson and match with top Python Tutors for concepts, projects and assignment help on Wiingy today!
Basic Input and Output in Python
The two most common functions for basic input and output in Python are input() and print(). The input() function is used to prompt the user for input, while the print() function is used to display output to the user.
Here is an example of using the input() function to prompt the user for their name and then using the print() function to display a greeting:
1name = input("What is your name? ")
2print("Hello, " + name + "!")
In this example, the input() function prompts the user for their name, and the value entered is assigned to the variable name. The print() function then displays a greeting message that includes the value of the name variable.
Here is another example of using the print() function to display output:
1print("The answer is", 42)
In this example, the print() function displays the text “The answer is” followed by the value 42. The comma between the two arguments separates them and automatically adds a space between them in the output.
You can also use string formatting to create more complex output. Here is an example using the format() method to insert a value into a string:
1age = 30
2print("I am {} years old".format(age))
In this example, the value of the age variable is inserted into the string using the curly braces {} as a placeholder. The format() method is then called on the string, with the value to be inserted passed as an argument.
These are just a few examples of basic input and output in Python using the input() and print() functions. In the next section, we will explore how to read and write files in Python.
Advanced Input Handling in Python
The input() function in Python has a few additional syntax and parameters that can be used for advanced input handling. The basic syntax of the input() function is as follows:
1input(prompt)
The prompt parameter is a string that is displayed to the user to prompt them for input.
The input() function can also be used to take different types of input, including numeric and string input, and handle multiple inputs in a single line. Here are a few examples:
1# Taking integer input
2age = int(input("What is your age? "))
3
4# Taking floating-point input
5temperature = float(input("What is the temperature? "))
6
7# Taking multiple inputs in a single line
8name, age, city = input("Enter your name, age, and city: ").split(", ")
In the first example, the int() function is used to convert the string input to an integer. In the second example, the float() function is used to convert the string input to a floating-point number. In the third example, the split() method is used to split the input string into multiple values, separated by a comma.
When handling input in Python, it is important to follow best practices, such as input validation and sanitization, meaningful prompts, and proper formatting of input data. Here is an example of validating user input to ensure that the input is a positive integer:
1while True:
2 try:
3 age = int(input("Enter your age: "))
4 if age < 0:
5 raise ValueError("Age must be a positive integer")
6 break
7 except ValueError:
8 print("Invalid input. Please enter a positive integer.")
In this example, the try and except statements are used to catch any errors that may occur when converting the input to an integer. The if statement checks that the input is a positive integer, and if not, a ValueError is raised with an appropriate error message.
Advanced Output Handling in Python
In addition to the basic print() function, Python provides several options for advanced output handling, including output formatting, alignment, and sign specification. The str.format() method is a powerful tool for formatting output in Python. Here are a few examples:
1# Formatting output by position
2print("{0} is a {1}".format("Python", "programming language"))
3
4# Formatting output by name
5print("{language} is a {type}".format(language="Python", type="programming language"))
6
7# Output alignment and sign specification
8print("{:<10} {:>10}".format("Left", "Right"))
9print("{:+d}".format(42))
In the first example, the 0 and 1 inside the curly braces {} correspond to the first and second arguments of the format() method, respectively. In the second example, the names language and type are used instead of indices to specify the values to be inserted into the string.
The third example shows how to align output to the left and right, and the fourth example shows how to specify a sign for numeric output.
Python also provides control sequences for modifying output and adding color to output. Here is an example of adding color to output:
1# Adding color to output
2print("\033[1;31;40m Red Text \n")
In this example, the control sequence \033[1;31;40m is used to set the text color to red. The sequence can be modified to change the color or other attributes of the text.
Python also provides options for outputting to files using the open() function and different file modes. Here is an example of writing output to a file:
1# Writing output to a file
2with open("output.txt", "w") as f:
3 f.write("Hello, world!")
In this example, the with statement is used to ensure that the file is properly closed after writing. The “w” parameter specifies that the file should be opened in write mode, and the write() method is used to write the output string to the file.
Python also supports reading input from files using the open() function and the read methods read(), readline(), and readlines(). Here is an example of reading input from a file:
1# Reading input from a file
2with open("input.txt", "r") as f:
3 for line in f:
4 print(line.strip())
In this example, the with statement is used to ensure that the file is properly closed after reading. The “r” parameter specifies that the file should be opened in read mode, and the for loop is used to iterate over each line of the file using the readline() method.
In conclusion, proper input and output handling is an essential aspect of programming in Python. Understanding the basic and advanced input and output functions, syntax, and parameters, as well as the best practices and options for input validation, sanitization, formatting, and output control, can help programmers create more efficient and effective Python programs.
Common Mistakes to Avoid
When using Python to handle input and output, programmers should avoid making a few common mistakes. They consist of:
- Not verifying input: It’s crucial to validate input to make sure it’s the right kind and falls within the expected range. If you don’t, your software can crash or produce inaccurate results.
- Sanitizing input: Input needs to be cleaned up to get rid of any malicious code or characters. Inaction on your part could lead to security flaws.
- Not formatting output: Output should be formatted correctly so that it is easy to read and understand.If this isn’t done, users may have trouble interpreting the results.
- Not closing files correctly: It’s important to close files correctly after reading from or writing to them to avoid memory leaks or file corruption.
- Best practices for managing input and output in Python include validating and sanitizing input, putting output in the right format, and making sure files are closed.
Python programming necessitates careful attention to managing input and output operations. While functions like
1input()
and 1print()
form the cornerstone of I/O handling, leveraging Python’s advanced capabilities—such as file handling, validation techniques, and output formatting—can significantly enhance the functionality and reliability of your programs.
By mastering input validation, sanitization, and proper file-handling techniques, programmers can ensure their code is secure, user-friendly, and efficient. These practices help prevent common mistakes, such as mishandling input types, overlooking sanitization, or improperly formatting output.
However, applying these concepts in real-world scenarios, such as assignments or projects, can be challenging. If you’re struggling with Python file handling in your assignments or need help managing advanced input/output operations, private tutoring can help you with Python assignments, offering a tailored approach to resolving these challenges and building a solid foundation.
Conclusion
Python programming requires careful management of input and output. Although the input() and print() functions are the fundamental building blocks of input and output (I/O) handling, Python programs may be made more effective and efficient by using a variety of extra features and choices.
Programmers can design programs that are simpler to use, more secure, and easier to comprehend by understanding the syntax and parameters of the input() and print() methods, as well as best practices for input validation, sanitization, formatting, and output control.
Looking to Learn Python? Book a Free Trial Lesson and match with top Python Tutors for concepts, projects and assignment help on Wiingy today!
FAQs
What is handling input?
Handling input is the process of dealing with the input devices such as keyboard, mouse, scanner, microphone, and touch screen to interact with the computer or a program. It involves collecting and processing the data entered through these devices to produce an output. For instance, when a user types on the keyboard, the computer receives the input and processes it to display it on the screen or perform a specific action. Handling input requires knowledge of the input devices and their functionalities, and the ability to manage the input data effectively.
How do you manage input and output operations?
The management of input and output operations involves several strategies to optimize the performance of a computer system. Some of the techniques used to manage input and output operations include buffering, caching, and spooling. Buffering involves temporarily storing input or output data in a buffer memory to enable the system to handle large volumes of data efficiently. Caching involves storing frequently accessed data in a cache memory to reduce the time needed to access the data. Spooling involves storing output data in a queue to allow the system to process other tasks while the data is being printed or displayed.
What is output handling?
Output handling is the process of managing the output devices such as printers, monitors, and speakers to display or produce the output of a program or system. Output handling involves controlling the format and appearance of the output, such as font size, color, and alignment. The process of output handling also involves managing the output queue, which allows the system to process other tasks while waiting for the output to be produced. Effective output handling requires knowledge of the output devices and their functionalities, as well as the ability to manage the output data effectively.
What is input and output system in OS?
The input and output system in OS is responsible for managing the communication between the computer system and the input/output devices. It involves handling the input data from the input devices such as the keyboard, mouse, and touch screen, and managing the output data to the output devices such as the monitor, printer, and speakers. The input and output system in OS includes several components such as device drivers, input/output controllers, and input/output subsystems. These components work together to ensure that the input/output operations are properly managed, and the data is accurately processed, displayed, or stored. Effective management of the input and output system in OS requires knowledge of the hardware and software components of the computer system, as well as the ability to troubleshoot and resolve any input/output issues that may arise.
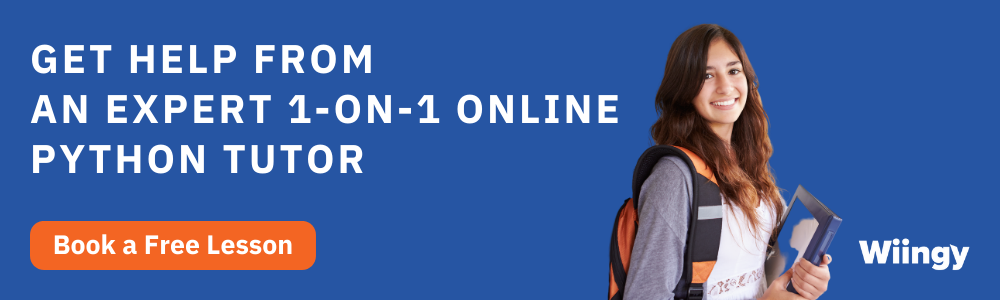
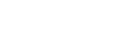
Jan 30, 2025
Was this helpful?