Python Tutorials
What are Ternary Operators in Python?
The ternary operator in Python is a shortcut for building a straightforward if-else statement. It is also known as a conditional expression, and it enables programmers to create code that is clear and legible. It is an important tool for Python programming because it streamlines the code and cuts down on the number of lines.
The ternary operator in Python is important because it makes it easy to make conditional statements. By putting the same reasoning on fewer lines, the code is easier to read and understand. By using fewer if-else lines, it also helps improve the way the code is run.
In this post, we’ll go over the ternary operator’s fundamental grammar and give examples of how to apply it to straightforward conditional statements.
Looking to Learn Python? Book a Free Trial Lesson and match with top Python Tutors for concepts, projects and assignment help on Wiingy today!
Simple Syntax for Ternary Operator
The syntax for the ternary operator in Python is as follows:
[on_true] if [expression] else [on_false]
Here, expression is a boolean expression, and on_true and on_false are the values that are returned based on the result of the boolean expression. If the expression is True, then the value of on_true is returned, else the value of on_false is returned.
Let’s look at some examples of how to use the ternary operator for simple conditional statements:
Example 1:
x = 10
y = 20
max_value = x if x > y else y
print(max_value)
Output:
20
In this example, we have used the ternary operator to check if x is greater than y. If the expression is True, then the value of x is returned, else the value of y is returned.
Example 2:
number = 12
even_odd = "Even" if number % 2 == 0 else "Odd"
print(even_odd)
Output:
Even
In this example, we have used the ternary operator to check if number is even or odd. If the expression number % 2 == 0 is True, then the value “Even” is returned, else the value “Odd” is returned.
Example 3:
is_raining = True
activity = "Stay indoors" if is_raining else "Go for a walk"
print(activity)
Output:
Stay indoors
In this example, we have used the ternary operator to check if it’s raining outside. If the expression is True, then the value “Stay indoors” is returned, else the value “Go for a walk” is returned.
Nested Ternary Operators
Nested ternary operators are a way to write more complex conditional statements using the ternary operator. A nested ternary operator is a ternary operator within another ternary operator. It allows us to write multiple conditions in a single line of code.
The syntax for a nested ternary operator is as follows:
[on_true] if [expression] else ([on_true] if [expression] else [on_false])
Here, the expression is evaluated first, and if it is true, the first on_true value is returned. If it is false, then the second expression is evaluated, and if it is true, the second on_true value is returned, else the on_false value is returned.
Let’s look at an example of how to use nested ternary operators in Python:
Example 1:
number = 50
result = "Greater than 50" if number > 50 else ("Equal to 50" if number == 50 else "Less than 50")
print(result)
Output:
Equal to 50
In this example, we have used a nested ternary operator to check if the number is greater than, equal to, or less than 50. If the number is greater than 50, then the value “Greater than 50” is returned. If it is equal to 50, then the value “Equal to 50” is returned. Else, the value “Less than 50” is returned.
Example 2:
pythonCopy code
age = 15
message = "Child" if age < 18 else ("Adult" if age >= 18 and age < 65 else "Senior")
print(message)
Output:
Child
In this example, we have used a nested ternary operator to check if age is a child, adult, or senior citizen. If age is less than 18, then the value “Child” is returned. If it is greater than or equal to 18 and less than 65, then the value “Adult” is returned. Else, the value “Senior” is returned.
Printing with Ternary Operator
We can also use the print() function within a ternary operator in Python. The syntax for using the print() function with a ternary operator is the same as the syntax for a regular ternary operator. We simply need to replace the on_true and on_false values with the print() function calls.
Let’s look at some examples of how to use the ternary operator with the print() function in Python:
Example 1:
age = 20
print("You are an adult" if age >= 18 else "You are a child")
Output:
You are an adult
In this example, we have used the ternary operator to print a message based on whether age is greater than or equal to 18.
Example 2:
number = 5
print("Even" if number % 2 == 0 else "Odd", "number")
Output:
Odd number
In this example, we have used the ternary operator to print whether number is even or odd along with the string “number”.
Ternary Operator Before Python 2.5
Before the introduction of the ternary operator in Python 2.5, programmers used a different method to write conditional expressions on a single line of code. This method involved using the if and else statements within parentheses to create a tuple.
Here is an example of how the conditional expression would be written before Python 2.5:
x = 5
result = (x + 1) if (x > 0) else (x - 1)
print(result)
Output:
6
In this example, we are checking whether x is greater than 0. If it is, then the value x + 1 is returned. Otherwise, the value x – 1 is returned. This is achieved using the if and else statements within parentheses.
However, this method is less readable and more confusing than the ternary operator. The ternary operator provides a clearer and more concise way to write conditional expressions on a single line of code.
Limitations of Ternary Operator
While the ternary operator is a useful tool for writing conditional expressions on a single line of code, it has some limitations that programmers should be aware of.
One limitation of the ternary operator is that it can make the code harder to read and understand, especially when nested ternary operators are used. In such cases, it is often better to use traditional if and else statements.
Another limitation is that the ternary operator can only handle simple expressions. Complex expressions involving multiple conditions or multiple operations should be avoided or written using traditional if and else statements.
Lastly, it is important to remember that the ternary operator should not be overused. It should only be used in cases where it improves the readability of the code and not just for the sake of writing code on a single line.
To overcome these limitations, it is best to follow some best practices when using the ternary operator in Python. These include:
- Limiting the use of nested ternary operators
- Using parentheses to clarify the order of operations
- Avoiding complex expressions
- Writing code that is easy to read and understand
- Using the ternary operator only when it improves the readability of the code
By following these best practices, programmers can effectively use the ternary operator in Python without compromising the readability and maintainability of their code.
Ternary Operator using Tuples
In addition to simple conditional statements, the ternary operator can also be used with tuples to return different values based on the condition.
Here is an example of how to use tuples with the ternary operator:
x = 5
y = 7
result = (x, y) if (x > y) else (y, x)
print(result)
Output:
(7, 5)
In this example, we are checking whether x is greater than y. If it is, then the tuple (x, y) is returned. Otherwise, the tuple (y, x) is returned.
Using tuples with the ternary operator allows for more complex conditional statements to be written on a single line of code.
Ternary Operator using Dictionaries
The ternary operator can also be used with dictionaries in Python. This allows for different values to be returned based on the condition.
Here is an example of how to use dictionaries with the ternary operator:
x = 5
y = 7
result = {'greater': x, 'lesser': y}[x > y]
print(result)
Output:
7
In this example, we are checking whether x is greater than y. If it is, then the value associated with the key ‘greater’ is returned from the dictionary. Otherwise, the value associated with the key ‘lesser’ is returned.
Using dictionaries with the ternary operator allows for more complex conditional statements to be written on a single line of code. It also provides more flexibility in returning different types of values based on the condition.
Ternary Operator using Lambdas
In Python, a lambda function is a small, anonymous function that can take any number of arguments, but can only have one expression. The ternary operator can be used with lambdas to create more concise and readable code.
Here is an example of how to use lambdas with the ternary operator:
x = 5
y = 7
result = (lambda: x if x > y else y)()
print(result)
Output:
7
In this example, we are using a lambda function that checks whether x is greater than y. If it is, then x is returned. Otherwise, y is returned. The () at the end of the lambda function call is necessary to execute the function and return its value.
Using lambdas with the ternary operator allows for more concise and readable code in situations where a simple conditional statement is needed.
Nested Ternary Operators
Nested ternary operators can be used to create more complex conditional statements in Python. A nested ternary operator consists of one or more ternary operators inside another ternary operator.
Here is an example of how to use nested ternary operators:
x = 5
y = 7
result = 'x > y' if x > y else ('x == y' if x == y else 'x < y')
print(result)
Output:
x < y
In this example, we are using a nested ternary operator to check whether x is greater than y, equal to y, or less than y. If x is greater than y, then the string ‘x > y’ is returned. If x is equal to y, then the string ‘x == y’ is returned. Otherwise, the string ‘x < y’ is returned.
Using nested ternary operators can make code more concise and readable in situations where multiple conditions need to be checked.
Conclusion
In conclusion, the ternary operator is an important feature in Python that allows for concise and readable code. It is especially useful in situations where simple conditional statements are needed. By using lambdas, tuples, dictionaries, and nested ternary operators with the ternary operator, programmers can write even more complex conditional statements in a readable and efficient way.
In this article, we have covered the basic syntax of the ternary operator, as well as more advanced topics such as nested ternary operators, printing with the ternary operator, and using lambdas, tuples, and dictionaries with the ternary operator. By mastering these concepts, programmers can write more efficient and readable code in real-world applications.
Looking to Learn Python? Book a Free Trial Lesson and match with top Python Tutors for concepts, projects and assignment help on Wiingy today!
FAQs
What are ternary operators in Python?
In Python, ternary operators are a way to write an if-else statement in a shorter format. They are operators that take three operands and evaluate a condition, then return one of two values depending on the result of the evaluation. Ternary operators are represented using the syntax: condition_if_true if condition else condition_if_false.
What is ternary operator with example?
One example of using a ternary operator in Python is to check if a number is positive or negative:
num = -5
result = “positive” if num >= 0 else “negative”
print(result)
In this example, the condition being evaluated is num >= 0, which checks if the number is non-negative. If the condition is true, the ternary operator returns the string “positive”, otherwise it returns the string “negative”. In this case, since num is negative, the result is “negative”.
What is ternary operator with 3 conditions in Python?
The ternary operator in Python only takes two conditions, so it’s not possible to create a ternary operator with three conditions directly. However, you can use nested ternary operators to achieve a similar effect. Here’s an example:
num = 7
result = "positive" if num > 0 else ("zero" if num == 0 else "negative")
print(result)
In this example, the first condition being evaluated is num > 0, which checks if the number is positive. If the condition is true, the ternary operator returns the string “positive”. If the first condition is false, the nested ternary operator is evaluated. The nested ternary operator checks if the numberis zero by evaluating num == 0. If the condition is true, the nested ternary operator returns the string “zero”. If both the first and nested conditions are false, the ternary operator returns the string “negative”. Although this technique involves using nested ternary operators, it can provide a way to achieve the functionality of a ternary operator with three conditions.
Does ternary operator exist in Python?
Yes, Python has a ternary operator, which is a shorthand for an if-else statement. The syntax for the ternary operator in Python is: condition_if_true if condition else condition_if_false. This operator is particularly useful when you want to write concise code that performs a quick conditional check and returns a value based on the result of the check.
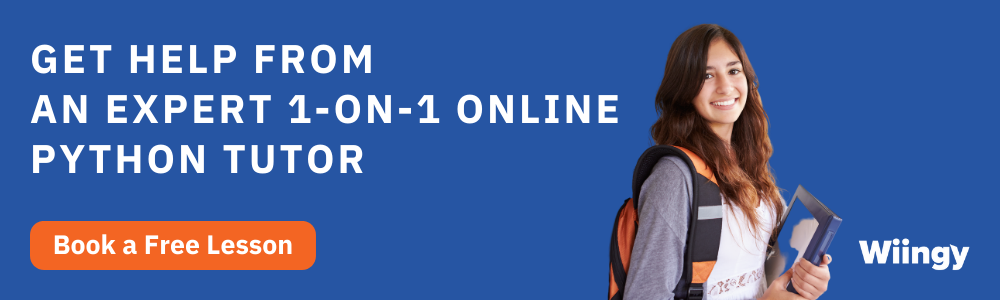
Written by by
Rahul LathReviewed by by
Arpit Rankwar