In this article
What are Python Tuples?
Creating and Accessing Tuples in Python
Delete Tuple Elements
Modifying Tuples in Python
Built-in Tuple Functions in Python
Tuple Comprehensions in Python
Indexing, Slicing, and Matrices in Python
Concatenation of Tuples in Python
Iterating through a Tuple in Python
Check if an Item Exists in the Python Tuple
Solved Examples
Advantages of Tuple over List in Python
Conclusion
FAQs
What are Python Tuples?
In Python, a tuple is a collection of ordered, immutable, and unique elements. Unlike lists, which are mutable, tuples cannot be modified once they are created. This makes them particularly useful in cases where you want to store data that should not be altered, such as constant values, dates, or coordinate points. The elements of a tuple are enclosed within round brackets () and are separated by commas.
A. Comparison with lists and dictionaries
Python tuples are similar to lists in that they are both ordered and can store any type of data. However, the main difference between the two is that lists are mutable, meaning their elements can be changed, whereas tuples are immutable, meaning their elements cannot be modified. On the other hand, dictionaries are unordered and store data as key-value pairs, while tuples store elements without any keys.
B. Uses and benefits of using tuples in Python
There are several reasons why you might choose to use tuples over lists or dictionaries in Python. For example:
- Tuples are faster than lists because they cannot be modified and require less overhead in terms of memory and processing power.
- Tuples can be used as keys in dictionaries, while lists cannot.
- Tuples provide a simple way to store and pass around multiple values without having to create a custom data structure.
- Tuples can be used to return multiple values from a function, making it easier to pass information between different parts of your code.
Looking to Learn Python? Book a Free Trial Lesson and match with top Python Tutors for concepts, projects and assignment help on Wiingy today!
Creating and Accessing Tuples in Python
A. Creating tuples using parentheses
To create a tuple in Python, you simply enclose a comma-separated list of values within parentheses. For example:
1my_tuple = (1, 2, 3)
2print(my_tuple)
3(1, 2, 3)
You can also create tuples containing different data types, such as integers, strings, and even other tuples:
1mixed_tuple = (1, "hello", (4, 5))
2 print(mixed_tuple)
3(1, "hello", (4, 5))
B. Accessing elements in a tuple using indices
To access an element in a tuple, you use square brackets [] and the index of the element you want to retrieve. For example:
1my_tuple = (1, 2, 3)
2 print(my_tuple[0])
31
Note that indices in Python start at 0, so to access the first element of a tuple, you would use the index 0, and so on.
C. Unpacking tuples into variables
In addition to accessing individual elements in a tuple, you can also unpack a tuple into separate variables. This allows you to assign the values in a tuple to multiple variables in a single line of code:
1my_tuple = (1, 2, 3)
2 a, b, c = my_tuple
3 print(a)
41
5 print(b)
62
7 print(c)
83
Unpacking tuples can be especially useful when working with functions that return multiple values, as it allows you to easily assign these values to separate variables for further processing.
Delete Tuple Elements
It is not possible to delete individual elements from a tuple, as tuples are immutable in Python. This means that once a tuple has been created, its elements cannot be changed or removed. If you need to modify the data in a tuple, you must create a new tuple with the desired elements.
However, you can delete an entire tuple by using the del statement:
1my_tuple = (1, 2, 3)
2 print(my_tuple)
3(1, 2, 3)
4 del my_tuple
5 print(my_tuple)
6NameError: name 'my_tuple' is not defined
In this example, the ‘del’ statement deletes the entire tuple, and trying to access the tuple afterwards results in a ‘NameError’, as the tuple no longer exists.
Modifying Tuples in Python
A. Explanation of why tuples are immutable
In Python, tuples are immutable, which means that once a tuple has been created, its elements cannot be changed. This is an intentional design decision in Python, as it allows for faster and more efficient processing of data, as well as making it easier to reason about the behavior of your code.
B. Workarounds for modifying tuples in Python
While you cannot modify the elements of a tuple directly, there are several workarounds that can be used to achieve similar results. For example:
Concatenating tuples: You can create a new tuple by concatenating two or more existing tuples:
1my_tuple = (1, 2, 3)
2 my_new_tuple = my_tuple + (4, 5, 6)
3 print(my_new_tuple)
4(1, 2, 3, 4, 5, 6)
Slicing tuples: You can create a new tuple by slicing an existing tuple:
1my_tuple = (1, 2, 3, 4, 5)
2 my_new_tuple = my_tuple[1:3]
3 print(my_new_tuple)
4(2, 3)
Converting tuples to lists: You can convert a tuple to a list, modify the list, and then convert it back to a tuple:
1my_tuple = (1, 2, 3)
2 my_list = list(my_tuple)
3 my_list.append(4)
4 my_new_tuple = tuple(my_list)
5 print(my_new_tuple)
6(1, 2, 3, 4)
C. Converting tuples to lists and back
As seen in the previous example, converting tuples to lists and back is a simple and effective way to modify the elements of a tuple in Python. The built-in list() and tuple() functions can be used to perform these conversions:
1my_tuple = (1, 2, 3)
2 my_list = list(my_tuple)
3 print(my_list)
4[1, 2, 3]
5 my_new_tuple = tuple(my_list)
6 print(my_new_tuple)
7(1, 2, 3
Built-in Tuple Functions in Python
Python provides several built-in functions for working with tuples, which can help you perform common operations and manipulations with ease.
A. len(), max(), min(), sum()
The len() function returns the number of elements in a tuple:
1my_tuple = (1, 2, 3)
2 print(len(my_tuple))
33
The max() and min() functions return the maximum and minimum elements in a tuple, respectively:
1my_tuple = (1, 2, 3)
2 print(max(my_tuple))
33
4 print(min(my_tuple))
51
The sum() function returns the sum of all elements in a tuple:
1my_tuple = (1, 2, 3)
2 print(sum(my_tuple))
36
B. sorted(), enumerate()
The sorted() function returns a sorted list of the elements in a tuple:
1my_tuple = (3, 2, 1)
2 print(sorted(my_tuple))
3[1, 2, 3]
The enumerate() function adds a counter to an iterable and returns it in a form of enumerate object, which can be used to iterate through elements of a tuple along with their index:
1my_tuple = (1, 2, 3)
2 for i, value in enumerate(my_tuple):
3... print(i, value)
4...
50 1
61 2
72 3
C. count(), index()
The count() function returns the number of occurrences of a specified element in a tuple:
1my_tuple = (1, 2, 3, 1, 2)
2 print(my_tuple.count(1))
32
The index() function returns the index of the first occurrence of a specified element in a tuple:
1my_tuple = (1, 2, 3, 1, 2)
2 print(my_tuple.index(2))
31
Tuple Comprehensions in Python
A. Explanation of Tuple Comprehensions
Tuple comprehensions are a concise and efficient way to create new tuples based on existing tuples in Python. They are similar to list comprehensions, but return a tuple instead of a list.
B. Syntax and Examples
The syntax of a tuple comprehension is as follows:
1new_tuple = (expression for item in iterable)
Where expression is a function or operation applied to each item in the iterable, and iterable is an existing tuple.
For example, to create a new tuple that contains the squares of the numbers in an existing tuple:
1my_tuple = (1, 2, 3)
2 new_tuple = (i**2 for i in my_tuple)
3 print(new_tuple)
4(1, 4, 9)
Tuple comprehensions can also be used with conditional statements to filter elements from the iterable:
1my_tuple = (1, 2, 3, 4, 5)
2 new_tuple = (i for i in my_tuple if i % 2 == 0)
3 print(new_tuple)
4(2, 4)
Indexing, Slicing, and Matrices in Python
A. Indexing in Tuples
Indexing in Python allows you to access a specific element within a tuple. To access an element in a tuple, you use the index of the element, which starts at 0 for the first element and increases by 1 for each subsequent element. For example:
1my_tuple = (1, 2, 3, 4, 5)
2 print(my_tuple[0])
31
4 print(my_tuple[3])
54
B. Slicing in Tuples
Slicing in Python allows you to extract a portion of a tuple by specifying a start and end index. The syntax for slicing is as follows:
1new_tuple = original_tuple[start:end]
Where start is the index of the first element in the slice, and end is the index of the first element that is not included in the slice. For example:
1my_tuple = (1, 2, 3, 4, 5)
2 new_tuple = my_tuple[1:3]
3 print(new_tuple)
4(2, 3)
C. Matrices in Python
A matrix is a 2-dimensional collection of elements, often numbers. In Python, matrices can be represented using nested lists or nested tuples. To access elements within a matrix, you can use indexing and slicing in a similar way to accessing elements in a tuple.
For example, to access the element in the second row and third column of a matrix:
1my_matrix = [(1, 2, 3), (4, 5, 6), (7, 8, 9)]
2 print(my_matrix[1][2])
36
Concatenation of Tuples in Python
A. Definition
Concatenation in Python refers to the operation of combining two or more tuples into a single tuple. This allows you to combine multiple tuples into a single, larger tuple.
B. Concatenating Tuples
To concatenate two tuples in Python, you can use the + operator:
1tuple1 = (1, 2, 3)
2 tuple2 = (4, 5, 6)
3 new_tuple = tuple1 + tuple2
4 print(new_tuple)
5(1, 2, 3, 4, 5, 6)
You can also concatenate more than two tuples using the + operator multiple times:
1tuple1 = (1, 2, 3)
2 tuple2 = (4, 5, 6)
3 tuple3 = (7, 8, 9)
4 new_tuple = tuple1 + tuple2 + tuple3
5 print(new_tuple)
6(1, 2, 3, 4, 5, 6, 7, 8, 9)
C. Considerations
It is important to note that once a tuple is created, it is immutable and cannot be modified. Therefore, to concatenate tuples, you must create a new tuple.
Iterating through a Tuple in Python
A. Definition
Iteration in Python refers to the process of looping through the elements in a data structure, such as a tuple. This allows you to perform a specific action on each element in the tuple.
B. Using a For Loop
The most common way to iterate through a tuple in Python is to use a for loop:
1my_tuple = (1, 2, 3, 4, 5)
2 for element in my_tuple:
3... print(element)
4...
51
62
73
84
95
In this example, the for loop is used to loop through each element in the tuple my_tuple. For each iteration of the loop, the current element is assigned to the variable element, and the code within the loop is executed.
C. Using the enumerate() Function
Another common method for iterating through a tuple is to use the enumerate() function:
1my_tuple = (1, 2, 3, 4, 5)
2 for index, element in enumerate(my_tuple):
3... print(index, element)
4...
50 1
61 2
72 3
83 4
94 5
In this example, the enumerate() function is used to loop through each element in the tuple my_tuple. The enumerate() function provides both the index and the element for each iteration of the loop. This is useful when you need to access both the index and the value of each element in the tuple.
Check if an Item Exists in the Python Tuple
A. Definition
Checking if an item exists in a Python tuple involves searching for a specific value within the tuple and determining whether it is present or not.
B. Using the in Operator
The simplest way to check if an item exists in a tuple is to use the in operator:
1my_tuple = (1, 2, 3, 4, 5)
2 3 in my_tuple
3True
4 6 in my_tuple
5False
In this example, the in operator is used to check if the value 3 exists in the tuple my_tuple. Since 3 is present in the tuple, the expression returns True. On the other hand, the value 6 is not present in the tuple, so the expression returns False.
C. Using the index() Method
Another way to check if an item exists in a tuple is to use the index() method:
1my_tuple = (1, 2, 3, 4, 5)
2 try:
3... my_tuple.index(3)
4... except ValueError:
5... print("Value not found")
6...
7 try:
8... my_tuple.index(6)
9... except ValueError:
10... print("Value not found")
11...
12Value not found
In this example, the index() method is used to search for the value 3 in the tuple my_tuple. If the value is present, the method returns its index in the tuple. If the value is not present, the method raises a ValueError. To handle this, we use a try-except block to catch the error and print a message indicating that the value was not found.
Solved Examples
Example 1: Creating and Accessing a Tuple
1# Creating a Tuple
2numbers = (1, 2, 3, 4, 5)
3
4# Accessing elements in a Tuple
5print(numbers[0]) # Output: 1
6print(numbers[-1]) # Output: 5
7
8# Unpacking a Tuple into variables
9a, b, c, d, e = numbers
10print(a, b, c, d, e) # Output: 1 2 3 4 5
Example 2: Modifying a Tuple
1# Converting a Tuple to a List
2numbers = (1, 2, 3, 4, 5)
3numbers = list(numbers)
4numbers[0] = 10
5numbers = tuple(numbers)
6print(numbers) # Output: (10, 2, 3, 4, 5)
Example 3: Built-in Tuple Functions
1# len() function
2numbers = (1, 2, 3, 4, 5)
3print(len(numbers)) # Output: 5
4
5# max() and min() functions
6print(max(numbers)) # Output: 5
7print(min(numbers)) # Output: 1
8
9# count() function
10numbers = (1, 2, 3, 4, 5, 2, 2)
11print(numbers.count(2)) # Output: 3
12
13# index() function
14print(numbers.index(3)) # Output: 2
Example 4: Tuple Comprehensions
1# Tuple Comprehension
2numbers = (1, 2, 3, 4, 5)
3squared_numbers = tuple(x**2 for x in numbers)
4print(squared_numbers) # Output: (1, 4, 9, 16, 25)
Example 5: Concatenating Tuples
1# Concatenating Tuples
2tuple1 = (1, 2, 3)
3tuple2 = (4, 5, 6)
4tuple3 = tuple1 + tuple2
5print(tuple3) # Output: (1, 2, 3, 4, 5, 6)
Example 6: Iterating Through a Tuple
1# Iterating Through a Tuple
2numbers = (1, 2, 3, 4, 5)
3for number in numbers:
4 print(number)
5# Output:
6# 1
7# 2
8# 3
9# 4
10# 5
Example 7: Check if an Item Exists in the Tuple
1# Check if an Item Exists in the Tuple
2numbers = (1, 2, 3, 4, 5)
3if 3 in numbers:
4 print("3 exists in the Tuple")
5else:
6 print("3 does not exist in the Tuple")
7# Output: 3 exists in the Tuple
Advantages of Tuple over List in Python
A. Immutability
One of the main advantages of tuples over lists in Python is that tuples are immutable, meaning their elements cannot be changed after they are created. This makes tuples ideal for representing fixed and unchanging data, such as dates, times, or configuration settings. Since tuples are immutable, they are also safer to use in concurrent or multi-threaded environments where multiple processes may be accessing the same data simultaneously.
B. Performance
Tuples are also faster and use less memory than lists. This is because tuples are stored in a single block of memory, which allows the Python interpreter to access their elements more quickly. On the other hand, lists are implemented as dynamic arrays, which means they are stored in multiple blocks of memory and may require additional processing time to access their elements.
C. Readability
Tuples also have a more readable and concise syntax compared to lists. When using tuples, it is clear that the data they represent is meant to be fixed and unchanging, which makes the code easier to understand and maintain. Additionally, tuples can be used as keys in dictionaries, while lists cannot, which allows you to use tuples to represent complex data structures in a more organized and readable way.
Conclusion
In this article, we have explored the basics of Python tuples, including how to create and access elements, perform various operations, and take advantage of built-in functions. We have also compared tuples with lists and dictionaries, and discussed the advantages of using tuples in your code.
Tuples are a versatile data structure in Python, and understanding how to use them effectively can greatly enhance your ability to write efficient, readable, and maintainable code. Whether you are a beginner or an experienced Python programmer, incorporating tuples into your workflow is a valuable skill to have.
Looking to Learn Python? Book a Free Trial Lesson and match with top Python Tutors for concepts, projects and assignment help on Wiingy today!
FAQs
What is the difference between a list and a tuple in Python?
A tuple is similar to a list in Python, but there are a few key differences between the two. The main difference is that a tuple is immutable, meaning its elements cannot be changed once it has been created, whereas a list is mutable and its elements can be changed. Tuples use parentheses to define the elements, while lists use square brackets. Another difference is that tuples are typically used to store related data that belongs together, while lists are more flexible and can store any data type.
What is the difference between a list and a tuple in Python?
There are several reasons why you might choose to use a tuple instead of a list in Python. First, tuples are more efficient in terms of memory usage and execution time, as they are immutable and do not require the same level of overhead as lists. Additionally, because tuples are immutable, they can be used as key values in dictionaries, which is not possible with lists. Finally, tuples can provide better readability and structure to your code, making it easier to understand what data is being stored and how it is related
Can tuples be modified in Python? If not, how do you change their values?
Tuples in Python are immutable, which means that once they are created, their elements cannot be changed. However, there are several workarounds for modifying tuples in Python. For example, you can convert a tuple to a list, make the desired changes, and then convert the list back to a tuple. Another option is to create a new tuple that contains the modified elements, while leaving the original tuple unchanged.
How do you access elements in a tuple in Python?
To access elements in a tuple, you can use an index or a slice. An index is an integer value that represents the position of the element you want to access, while a slice is a range of elements. To access an element using an index, you use square brackets with the index value inside, like this: tuple_name[index]. To access a range of elements using a slice, you use square brackets with two index values separated by a colon, like this: tuple_name[start:end].
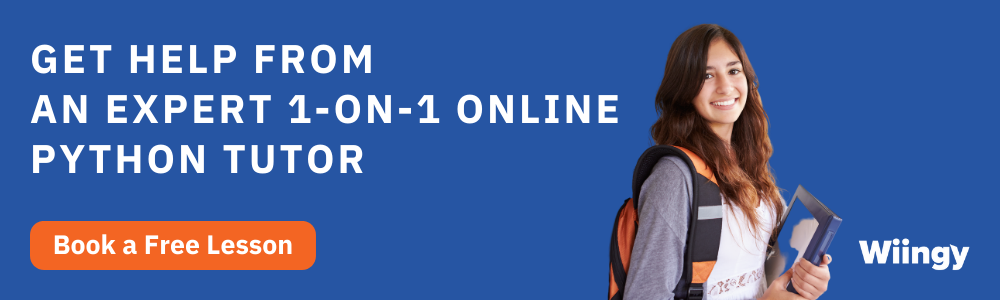
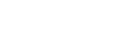
Jan 30, 2025
Was this helpful?