In Python, the “break” command is a control statement that can be used to end a loop early. A loop immediately stops running whenever a break statement is encountered inside of it, and program control is then passed to the statement that follows the loop.
When a programmer needs to exit a loop early due to a specific condition, the break statement is a crucial tool. It makes the code more readable and understandable and aids in increasing its efficiency. Programmers can use the break statement in a variety of situations, such as looking for a specific item in a list or ending a loop after a predetermined number of iterations.
Syntax of the Break Statement:
The basic syntax of the break statement is as follows:
1while condition: # loop body
2if some_condition: break
3# continue loop body
The above syntax is for a “while” loop, but it can also be used for “for” loops.
Example:
1# Program to search for an item in a list and exit the loop when found my_list = [1, 2, 3, 4, 5, 6] item_to_find = 4 for item in my_list: if item == item_to_find: print("Item found") break else: print("Item not found")
2# Output: Item found
Looking to Learn Python? Book a Free Trial Lesson and match with top Python Tutors for concepts, projects and assignment help on Wiingy today!
How does the Break Statement in Python work?
Python break statement works by prematurely exiting a loop based on a certain condition. When the break statement is encountered inside a loop, the loop immediately stops executing, and the program control is transferred to the statement that comes immediately after the loop.
A. Understanding the role of the Break Statement in loops:
The break statement is used in loops such as “for” and “while” to exit or terminate the loop early based on a certain condition. The loop continues to execute until the break statement is encountered, and then the loop is terminated.
B. Examples of using the Break Statement:
1/code start/ # Program to terminate a loop after a certain number of iterations
2for i in range(1, 11):
3if i == 6:
4break
5print(i)
6# Output: 1 2 3 4 5
7
8# Program to terminate a loop when a condition is met
9
10my_list = [10, 20, 30, 40, 50] condition = 25
11for item in my_list: if item == condition:
12print("Condition met") break else:
13print("Condition not met")
14# Output: Condition not met Condition not met Condition met
Types of Loops that can use Break Statement:
A. For Loops:
In “for” loops, the break statement can be used to end the loop early based on a predetermined condition. When looking for a particular value in a list or when the loop needs to end after a certain number of iterations, this is helpful.
Example:
1# Program to exit a for loop when the item is found in the list my_list = [10, 20, 30, 40, 50] item_to_find = 30 for item in my_list: if item == item_to_find: print("Item found") break else: print("Item not found")
2# Output: Item not found Item not found Item found
B. While Loops:
In “while” loops, the break statement can also be used to end the loop early based on a predetermined condition. This is helpful when the loop must end when a specific value is reached or a specific condition is satisfied.
Example:
1# Program to exit a while loop when the counter is greater than 5 counter = 1 while counter <= 10: print(counter) if counter > 5: break counter += 1
2# Output: 1 2 3 4 5 6
Real-World Applications of Break Statement in Python
A. Finding a specific value in a list:
When a particular value is located in a list, the break statement can be used to end a loop immediately. This can be helpful in saving processing time and memory when looking for something in a big dataset.
Example:
1# Program to find the first occurrence of a specific value in a list my_list = [2, 3, 4, 1, 5, 6, 7, 8] value_to_find = 5 for index, value in enumerate(my_list): if value == value_to_find: print("Value found at index:", index) break
2 # Output: Value found at index: 4
B. Exiting a Program:
The break statement can be used to exit a program prematurely. This can be useful in situations where the program has completed its task or when an error occurs that requires the program to stop immediately.
Example:
1# Program to exit the program when the user enters "quit" while True: user_input = input("Enter a value: ") if user_input == "quit": break print("You entered:", user_input)
2# Output: Enter a value: hello You entered: hello Enter a value: quit
C. Stopping an infinite loop:
A loop that never ends can be broken using the break statement. The break statement can be used to stop an infinite loop based on a specific condition. An infinite loop is a loop that runs indefinitely.
Example:
1# Program to exit an infinite loop when the user enters "quit" while True: user_input = input("Enter a value: ") if user_input == "quit": break print("You entered:", user_input)
2 # Output: Enter a value: hello You entered: hello Enter a value: quit
Advantages of Break Statement in Python
- The break statement can save processing time and memory by exiting a loop as soon as a certain condition is met.
- It can help to make the code more readable and understandable by reducing unnecessary iterations.
- The break statement can be used to handle unexpected situations, such as terminating a program or stopping an infinite loop.
Conclusion
This blog post covered the Python break statement, including its significance, syntax, and applications in different kinds of loops. We also looked at some practical uses for the break statement, along with some of its benefits and drawbacks.
A helpful control statement that can increase the effectiveness and readability of code is the break statement. It can be used to prematurely end a loop based on a condition in a variety of programming scenarios. To prevent any unintended consequences, the break statement should be used carefully.
It is advised to practice coding exercises and investigate various programming scenarios in order to become an expert in the use of the break statement and other control statements in Python. This will enable a better understanding of when to use the break statement and how to do so efficiently.
Looking to Learn Python? Book a Free Trial Lesson and match with top Python Tutors for concepts, projects and assignment help on Wiingy today!
FAQs
How to break loop in Python?
To end a loop in Python, use the “break” statement. When a loop encounters the break statement, the loop immediately terminates and the control of the program is passed to the statement that follows the loop.
How do you write a break condition in Python?
To write a break condition in Python, you need to use the “if” statement to check for a specific condition. If the condition is met, the “break” statement is used to exit the loop. Here’s an example:
for i in range(1, 11): if i == 5: break print(i)
What is break statement with an example?
The break statement is a control statement in Python that is used to exit or terminate a loop prematurely based on a certain condition. Here’s an example:
for i in range(1, 11): if i == 5: break print(i)
This program will print the numbers 1 to 4 and then exit the loop when the value of “i” is equal to 5.
How do you break a statement in Python 3?
To break a statement in Python 3, you need to use the “break” keyword followed by a semicolon. Here’s an example:
for i in range(1, 11): if i == 5: break; print(i)
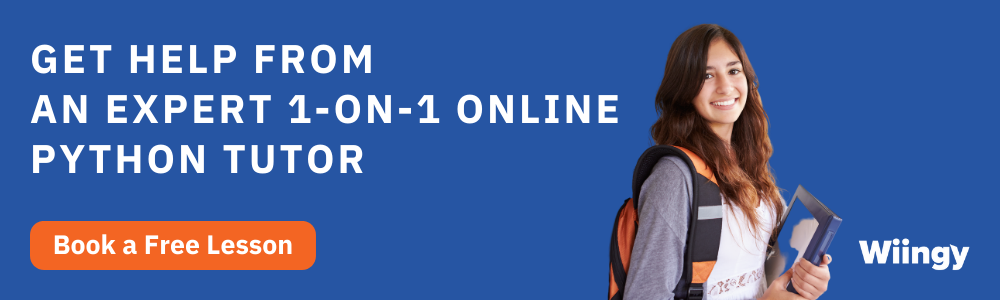
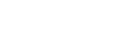
Jan 30, 2025
Was this helpful?