What is Python Lambda?
A lambda function in Python is a brief anonymous function with a single expression and unlimited number of arguments. It is called anonymous because it doesn’t have a name like a normal function, and it is defined using the keyword “lambda”.
Lambda functions are primarily used to build compact functions that can be passed as arguments to higher-order functions like map(), filter(), and reduce(). When a function is only required briefly and a named function does not need to be defined, lambda functions are also used.
Python lambda functions are crucial because they help make code more readable and concise. They also help to reduce the amount of code that needs to be written.
Looking to Learn Python? Book a Free Trial Lesson and match with top Python Tutors for concepts, projects and assignment help on Wiingy today!
Syntax of Python Lambda functions
The syntax of a lambda function is quite simple. It consists of the keyword “lambda”, followed by the arguments, a colon, and the expression. The general syntax of a lambda function is as follows:
1lambda arguments: expression
Code example of a simple lambda function:
Here is an example of a simple lambda function that adds two numbers:
1add = lambda x, y: x + y print(add(2, 3)) # Output: 5
In the above example, the lambda function takes two arguments x and y, and returns their sum.
How Python Lambda functions work
Anonymous functions, or functions without a name, are created using lambda functions. When a function is only required temporarily and a named function does not need to be defined, anonymous functions can be useful.
A. Code example of a lambda function in action:
Here is an example of a lambda function being used with the built-in map() function to square a list of numbers:
1numbers = [1, 2, 3, 4, 5] squared = list(map(lambda x: x ** 2, numbers)) print(squared) # Output: [1, 4, 9, 16, 25]
In the above example, the lambda function takes one argument x, and returns its square. The map() function applies the lambda function to each element in the numbers list, and returns a new list with the squared values.
B. Comparison with regular functions:
Lambda functions can take arguments and return values, just like regular functions can. The main difference is that lambda functions are anonymous and are defined using the keyword “lambda”. Regular functions, on the other hand, are named and are defined using the keyword “def”.
Here is an example of a regular function that adds two numbers:
1def add(x, y): return x + y print(add(2, 3)) # Output: 5
In this example, the add() function takes two arguments x and y, and returns their sum. The syntax is slightly different from the lambda function, but the result is the same.
Use cases of lambda functions
A. Simplifying code and reducing function definitions:
Code can be made simpler and with fewer function definitions by using lambda functions. To calculate the square of a number, for instance, a lambda function can be used rather than defining a separate function:
1square = lambda x: x ** 2 print(square(4)) # Output: 16
B. Using lambda functions in higher-order functions:
Higher-order functions like map(), filter(), and reduce() can take lambda functions as arguments. For example, a lambda function can be used with map() to convert a list of strings to uppercase:
1strings = ['apple', 'banana', 'cherry'] uppercase = list(map(lambda x: x.upper(), strings)) print(uppercase) # Output: ['APPLE', 'BANANA', 'CHERRY']
C. Creating inline functions for quick calculations:
Inline functions for quick calculations can be made using lambda functions. For example, a lambda function can be used with sorted() to sort a list of tuples by the second item:
1tuples = [('John', 50), ('Alice', 30), ('Bob', 40)] sorted_tuples = sorted(tuples, key=lambda x: x[1]) print(sorted_tuples) # Output: [('Alice', 30), ('Bob', 40), ('John', 50)]
D. Code examples of lambda functions in different scenarios:
Lambda functions can be used in various scenarios, such as sorting, filtering, and mapping lists, as well as in GUI programming and web development. Here are a few examples:
1# Sorting a list of dictionaries by a specific key people = [{'name': 'John', 'age': 30}, {'name': 'Alice', 'age': 20}, {'name': 'Bob', 'age': 40}] sorted_people = sorted(people, key=lambda x: x['age']) print(sorted_people) # Output: [{'name': 'Alice', 'age': 20}, {'name': 'John', 'age': 30}, {'name': 'Bob', 'age': 40}] # Filtering a list of even numbers numbers = [1, 2, 3, 4, 5, 6] even_numbers = list(filter(lambda x: x % 2 == 0, numbers)) print(even_numbers) # Output: [2, 4, 6] # Mapping a list of numbers to their squares numbers = [1, 2, 3, 4, 5] squares = list(map(lambda x: x ** 2, numbers)) print(squares) # Output: [1, 4, 9, 16, 25]
Limitations of lambda functions
A. Difficulty in debugging and testing lambda functions:
Since lambda functions are anonymous and don’t have names, it can be hard to find bugs and test them. This can make it challenging to isolate and fix errors in the code.
B. Inability to use statements and multiple expressions in lambda functions:
Lambda functions can only have one expression. They can’t include statements or more than one expression. This can make it hard for lambda functions to do complicated tasks.
C. Code examples of lambda function misuse:
Here are a few examples of lambda function misuse:
1/code start /# Incorrect use of a lambda function with multiple expressions invalid = lambda x, y: x + y; print(invalid(2, 3)) # Output: 5 # Incorrect use of a lambda function with a statement invalid = lambda x: print(x); invalid('hello') # Output: None
Conclusion
A. Summary of the importance and limitations of lambda functions:
In Python programming, lambda functions are a helpful tool that enable cleaner code, faster computations, and more flexibility in higher-order functions. However, they also have drawbacks, such as a lack of support for statements and multiple expressions and difficulties in testing and debugging.
B. Recommendations for using lambda functions effectively and avoiding common mistakes:
It’s crucial to keep lambda functions straightforward and keep them to single expressions if you want to use them effectively. Additionally, they ought to be applied sparingly and only in circumstances where they clearly outperform standard functions. To avoid errors and ensure proper operation, lambda functions must be thoroughly tested and debugged.
By understanding the uses and limitations of lambda functions, students can develop their Python programming skills and improve their code efficiency and readability.
Looking to Learn Python? Book a Free Trial Lesson and match with top Python Tutors for concepts, projects and assignment help on Wiingy today!
FAQs
What is lambda in Python?
In Python, Lambda is a keyword used to define anonymous functions, which are functions that do not have a name. Lambda functions are defined using a single line of code and are typically used to simplify code or create inline functions for quick calculations.
What is the use of lambda function?
The main use of lambda functions in Python is to create small, single-expression functions that can be used as arguments for higher-order functions or in other situations where a named function is not required. Lambda functions are often used to simplify code and reduce the number of function definitions required.
What is the benefit of lambda function in Python?
The benefit of using lambda functions in Python is that they provide a quick and easy way to create small, single-expression functions without the need for a named function. This can simplify code and reduce the number of function definitions required, making code more efficient and easier to read.
Why is it called lambda Python?
The name “lambda” comes from lambda calculus, a branch of mathematics that deals with functions and their behavior. In Python, the lambda keyword is used to define anonymous functions, which are similar to lambda functions in lambda calculus. The name “lambda” was adopted by the designers of Python as a nod to this mathematical background.
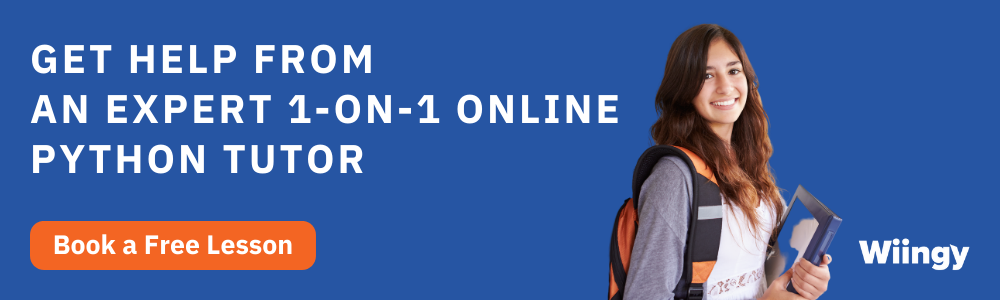
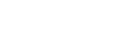
Jan 30, 2025
Was this helpful?