Python Tutorials
Conditional statements in Python are used to only run a specific code block when a predetermined condition is satisfied. According to how the conditions turn out, the program will take different paths.
Any circumstance that yields a True or False Boolean value qualifies as the condition. When the condition is True, a specific block of code is executed. The program either advances to the following block of code or skips it entirely when the condition is False. In Python, conditional statements are achieved using if, else, and elif statements.
Without conditional statements, programs would execute consistently regardless of the input or the state of the program at any given time.
Looking to Learn Python? Book a Free Trial Lesson and match with top Python Tutors for concepts, projects and assignment help on Wiingy today!
The If Statement:
The most fundamental conditional statement in Python is the if statement. It permits a particular block of code to be executed by the program only when a particular condition is satisfied.
A. Syntax of the if statement:
The syntax of the if statement in Python is as follows:
if condition: code block
The “if” keyword is followed by a condition that returns a Boolean value – either True or False. If the condition is True, then the code block is executed. If the condition is False, then the code block is skipped entirely.
B. How the if statement works:
The provided condition is evaluated by the if statement to determine how to proceed. The code block is executed if the condition is True. If the condition is False, then the code block is skipped entirely. This means that the program will follow different paths depending on the outcome of the condition.
C. Examples of using the if statement:
Example 1:
x = 10 if x > 5: print("x is greater than 5")
In this example, the condition “x > 5” is True because x is equal to 10, which is greater than 5. Therefore, the code block “print(‘x is greater than 5’)” is executed, and the output is “x is greater than 5”.
Example 2:
x = 2 if x > 5: print("x is greater than 5")
In this example, the condition “x > 5” is False because x is equal to 2, which is not greater than 5. Therefore, the code block “print(‘x is greater than 5’)” is skipped entirely, and there is no output.
Example 3:
age = 12 if age < 18: print("You are not old enough to vote.")
In this example, the condition “age < 18” is True because age is equal to 12, which is less than 18. Therefore, the code block “print(‘You are not old enough to vote.’)” is executed, and the output is “You are not old enough to vote.”
The If-Else Statement:
A more sophisticated conditional statement in Python is the if-else statement. It enables the program to run one code block when a particular condition is satisfied and another when the condition is not.
A. Syntax of the if-else statement:
The syntax of the if-else statement in Python is as follows:
if condition: code block 1 else: code block 2
The “if” keyword is followed by a condition that returns a Boolean value – either True or False. If the condition is True, then the code block 1 is executed. If the condition is False, then the code block 2 is executed.
B. How the if-else statement works:
The if-else statement works by evaluating the condition that is provided. If the condition is True, then the code block 1 is executed. If the condition is False, then the code block 2 is executed. This means that the program will follow different paths depending on the outcome of the condition.
C. Examples of using the if-else statement:
Example 1:
x = 10 if x > 5: print("x is greater than 5") else: print("x is less than or equal to 5")
In this example, the condition “x > 5” is True because x is equal to 10, which is greater than 5. Therefore, the code block “print(‘x is greater than 5’)” is executed, and the output is “x is greater than 5”.
Example 2:
x = 2 if x > 5: print("x is greater than 5") else: print("x is less than or equal to 5")
In this example, the condition “x > 5” is False because x is equal to 2, which is not greater than 5. Therefore, the code block “print(‘x is less than or equal to 5’)” is executed, and the output is “x is less than or equal to 5”.
The Elif Statement:
Another sophisticated conditional statement in Python is the elif statement. It enables the program to assess various conditions and run various code blocks based on which condition is satisfied.
A. Syntax of the elif statement:
The syntax of the elif statement in Python is as follows:
if condition 1:
code block 1
elif condition 2:
code block 2
else:
code block 3
The “if” keyword is followed by a condition that returns a Boolean value – either True or False. If the condition is True, then the code block 1 is executed. If the condition is False, then the program evaluates the next condition (condition 2). If condition 2 is True, then the code block 2 is executed. If condition 2 is False, then the code block 3 is executed.
B. How the elif statement works:
The elif statement works by evaluating multiple conditions in sequence until one of them is True. If a condition is True, then the corresponding code block is executed, and the program skips the remaining conditions and code blocks.
C. Examples of using the elif statement:
Example 1:
x = 10 if x > 10: print("x is greater than 10") elif x == 10: print("x is equal to 10") else: print("x is less than 10")
In this example, the condition “x > 10” is False because x is equal to 10. The condition “x == 10” is True, therefore, the code block “print(‘x is equal to 10’)” is executed, and the output is “x is equal to 10”.
Example 2:
age = 25 if age < 18: print("You are not old enough to vote.") elif age < 21: print("You can vote but cannot drink alcohol.") else: print("You can vote and drink alcohol.")
In this example, the program evaluates the conditions in sequence. The first condition “age < 18” is False because age is equal to 25. The second condition “age < 21” is also False because age is greater than or equal to 21. Therefore, the code block “print(‘You can vote and drink alcohol.’)” is executed, and the output is “You can vote and drink alcohol.”.
Nested Conditional Statements:
Use of conditional statements inside of other conditional statements is known as nesting. This allows for even more complex decision-making in a program.
A. Syntax of nested conditional statements:
The syntax of nested conditional statements in Python is as follows:
if condition1:
code block 1
if condition2:
code block 2
else:
code block 3
else:
code block 4
In this example, there is an if statement inside another if statement. The outer if statement is followed by a code block, which contains another if statement. Depending on the outcome of the nested if statement, the program can execute code block 2 or code block 3.
B. How nested conditional statements work:
The way nested conditional statements operate is by ranking the conditions. If the condition in the outer if statement is True, the program then evaluates the nested if statement. The corresponding code block is executed if the nested if statement is also True. If the nested if statement is False, then the program executes the else block.
C. Examples of using nested conditional statements:
Example 1:
x = 10
y = 5
if x > 5:
print("x is greater than 5")
if y > 2:
print("y is greater than 2")
else:
print("y is less than or equal to 2")
else:
print("x is less than or equal to 5")
In this example, the outer if statement is True because x is equal to 10, which is greater than 5. Therefore, the code block “print(‘x is greater than 5’)” is executed. The nested if statement is also True because y is equal to 5, which is greater than 2. Therefore, the code block “print(‘y is greater than 2’)” is also executed, and the output is “x is greater than 5” and “y is greater than 2”.
Example 2:
x = 3
y = 1
if x > 5:
print("x is greater than 5")
if y > 2:
print("y is greater than 2")
else:
print("y is less than or equal to 2")
else:
print("x is less than or equal to 5")
In this example, the outer if statement is False because x is equal to 3, which is not greater than 5. Therefore, the code block “print(‘x is less than or equal to 5’)” is executed, and the output is “x is less than or equal to 5”.
Logical Operators:
To combine multiple conditions, logical operators are used in conditional statements. The three logical operators in Python are and, or, and not.
A. Explanation of logical operators in Python:
The “and” operator returns True if both conditions are True. The “or” operator returns True if at least one of the conditions is True. The “not” operator reverses the outcome of a condition, so if the condition is True, then “not” returns False, and if the condition is False, then “not” returns True.
B. Examples of using logical operators in conditional statements:
Example 1:
x = 10
y = 5
if x > 5 and y > 2:
print("Both conditions are True")
else:
print("At least one condition is False")
In this example, both conditions “x > 5” and “y > 2” are True, so the code block “print(‘Both conditions are True’)” is executed, and the output is “Both conditions are True”.
Example 2:
x = 3
y = 1
if x > 5 or y > 2:
print("At least one condition is True")
else:
print("Both conditions are False")
In this example, neither condition “x > 5” nor “y > 2” is True, so the code block “print(‘Both conditions are False’)” is executed, and the output is “Both conditions are False”.
Example 3:
x = 10
if not x < 5:
print("The condition is True")
else:
print("The condition is False")
In this example, the condition “x < 5” is False because x is equal to 10, which is not less than 5. The “not” operator reverses the outcome of the condition, so the code block “print(‘The condition is True’)” is executed, and the output is “The condition is True”.
Short-circuit Evaluation
The logical operators in Python have a feature called short-circuit evaluation that makes it possible to evaluate complex conditions quickly.
A. Explanation of short-circuit evaluation in Python:
The program can evaluate the bare minimum set of conditions required to determine the result of a logical expression thanks to short-circuit evaluation. This means that the program does not evaluate the other conditions if the result of the expression can be determined by evaluating only the first condition. For instance, in an “and” expression, the program does not evaluate the second condition because, if the first condition is False, the entire expression must also be False.
B. Examples of using short-circuit evaluation in conditional statements:
Example 1:
x = 10 y = 0 if y != 0 and x/y > 5: print("The condition is True") else: print("The condition is False")
In this example, the condition “y != 0” is True because y is equal to 0. The “and” operator requires both conditions to be True, so the program does not evaluate the second condition “x/y > 5” because the entire expression must be False. Therefore, the code block “print(‘The condition is False’)” is executed, and the output is “The condition is False”.
Example 2:
x = 10 y = 5 if y != 0 and x/y > 5: print("The condition is True") else: print("The condition is False")
In this example, both conditions “y != 0” and “x/y > 5” are True, so the entire expression is True. Therefore, the code block “print(‘The condition is True’)” is executed, and the output is “The condition is True”.
Chaining Conditional Statements:
Multiple conditions can be combined using logical operators by chaining conditional statements.
A. Syntax of chaining conditional statements:
The syntax of chaining conditional statements in Python is as follows:
if condition1 and condition2 or condition3:
code block
In this example, the program evaluates the conditions in order. The “and” operator requires both condition1 and condition2 to be True, and the “or” operator requires at least one of condition2 or condition3 to be True. If the entire expression is True, then the code block is executed.
B. How chaining conditional statements work:
Using logical operators, chaining conditional statements evaluate the conditions sequentially. The program assesses each condition individually until it can determine how the entire expression will turn out.
C. Examples of using chained conditional statements:
Example 1:
x = 10
y = 5
z = 3
if x > y and y > z:
print("The conditions are True")
else:
print("The conditions are False")
In this example, both conditions “x > y” and “y > z” are True, so the entire expression is True. Therefore, the code block “print(‘The conditions are True’)” is executed, and the output is “The conditions are True”.
Example 2:
x = 10
y = 5
z = 15
if x > y and y > z:
print("The conditions are True")
else:
print("The conditions are False")
In this example, the first condition “x > y” is True, but the second condition “y > z” is False. Therefore, the entire expression is False, and the code block “print(‘The conditions are False’)” is executed, and the output is “The conditions are False”.
Ternary Operator
The ternary operator is a shorthand way to write an if-else statement in Python.
A. Syntax of the ternary operator:
The syntax of the ternary operator in Python is as follows:
value_if_true
if condition
else
value_if_false
In this example, the program evaluates the condition. If the condition is True, then the expression returns the value “value_if_true”. If the condition is False, then the expression returns the value “value_if_false”.
B. How the ternary operator works:
The ternary operator works by evaluating the condition and returning one of two possible values, depending on the outcome of the condition. It is a more concise way to write an if-else statement.
C. Examples of using the ternary operator:
Example 1:
x = 10
result = "x is greater than 5"
if x > 5
else
"x is less than or equal to 5"
print(result)
In this example, the ternary operator evaluates the condition “x > 5”. Because x is equal to 10, which is greater than 5, the expression returns the value “x is greater than 5”. Therefore, the output is “x is greater than 5”.
Example 2:
x = 2
result = "x is greater than 5"
if x > 5
else
"x is less than or equal to 5"
print(result)
In this example, the ternary operator evaluates the condition “x > 5”. Because x is equal to 2, which is not greater than 5, the expression returns the value “x is less than or equal to 5”. Therefore, the output is “x is less than or equal to 5”.
Conclusion
In conclusion, Python’s if-else and elif statements are effective tools for managing a program’s flow. Depending on how a condition turns out, they enable the execution of various code blocks. Nested conditional statements and logical operators can be used to create even more complex decision-making in a program. The ternary operator provides a shorthand way to write an if-else statement.
Programming requires the use of conditional statements. They enable a program to decide based on the information provided as input and the desired result. Programmers can create more effective, flexible code that can handle a variety of situations by using conditional statements. Understanding how to use conditional statements in Python is a key skill for any programmer to have.
Looking to Learn Python? Book a Free Trial Lesson and match with top Python Tutors for concepts, projects and assignment help on Wiingy today!
FAQs
What is Elif and if-else Python?
if-else and elif are conditional statements in Python that are used to control the flow of the program. if-else statements are used to execute different code blocks based on the outcome of a condition. elif statements are used to add additional conditions to an if-else block.
What is the example of if Elif in Python?
Here is an example of using if-elif in Python
age = 25
if age < 18: print(“You are not old enough to vote.”)
elif age < 21: print(“You can vote but cannot drink alcohol.”)
else: print(“You can vote and drink alcohol.”)
In this example, the program evaluates the conditions in sequence and executes the corresponding code block based on the outcome of the condition.
What are the 3 statements in Python?
The 3 statements in Python are:
if statement
for statement
while statement
How can I use if-else in Python?
The true and false parts of a given condition are both executed using the if-else statement. If the condition is true, the if block code is executed and if the condition is false, the else block code is executed.
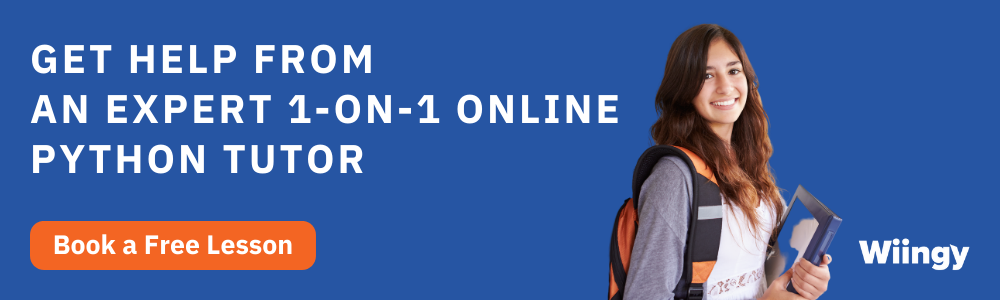
Written by by
Rahul LathReviewed by by
Arpit Rankwar