What are Operator Functions in Python
Operator functions in Python are built-in operations that operate on two or more operands. Basic mathematical operations, including addition, subtraction, multiplication, division, and others, are carried out using these operator functions.
Python operator functions are very important in programming because they make it easy and quick to do math calculations.Without them, programmers would have to manually write each piece of code, which would take time and increase the likelihood of mistakes.
The most popular operator functions in Python will be covered in this blog article, along with examples and explanations for each. Readers should have a better understanding of how to use operator functions in their Python scripts by the end of this article.
Looking to Learn Python? Book a Free Trial Lesson and match with top Python Tutors for concepts, projects and assignment help on Wiingy today!
List of Common Operator Functions in Python
A. add(a, b) – The add() function returns the sum of two numbers, a and b. This function can be used with any numeric data type in Python, such as integers, floats, or complex numbers.
1a = 5
2b = 10
3c = complex(2, 3)
4
5print("a + b =", add(a, b))
6print("b + c =", add(b, c))
7
8Output:
9a + b = 15
10b + c = (12+3j)
B. sub(a, b) The sub() function returns the difference between two numbers, a and b.
1a = 20
2b = 5
3
4print("a - b =", sub(a, b))
5
6Output:
7a - b = 15
C. mul(a, b) The mul() function returns the product of two numbers, a and b.
1a = 3
2b = 4
3
4print("a * b =", mul(a, b))
5
6Output:
7a * b = 12
D. truediv(a,b) The truediv() function returns the quotient of two numbers, a and b. This function performs true division, which means that the result is always a float, even if the operands are integers.
1a = 10
2b = 2
3
4print("a / b =", truediv(a, b))
5
6Output:
7a / b = 5.0
E. floordiv(a,b) The floordiv() function returns the quotient of two numbers, a and b, rounded down to the nearest integer. This function performs floor division, which means that the result is always an integer.
1a = 10
2b = 3
3
4print("a // b =", floordiv(a, b))
5
6Output:
7a // b = 3
F. pow(a,b) The pow() function returns the result of raising a to the power of b.
1a = 2
2b = 3
3
4print("a ** b =", pow(a, b))
5
6Output:
7a ** b = 8
G. mod(a,b) The mod() function returns the remainder of dividing a by b.
1a = 15
2b = 4
3
4print("a % b =", mod(a, b))
5
6Output:
7a % b = 3
H. lt(a, b) The lt() function returns True if a is less than b, otherwise, it returns False.
1a = 5
2b = 10
3
4print("a < b is", lt(a, b))
5
6Output:
7a < b is True
I. le(a, b) The le() function returns True if a is less than or equal to b, otherwise, it returns False.
1a = 5
2b = 5
3
4print("a <= b is", le(a, b))
5
6Output:
7a <= b is True
J. eq(a, b) The eq() function returns True if a is equal to b, otherwise, it returns False.
1a = 5
2b = 10
3
4print("a == b is", eq(a, b))
5
6Output:
7a == b is False
K. gt(a, b) The gt() function returns True if a is greater than b, otherwise, it returns False.
1a = 10
2b = 5
3
4print("a > b is", gt(a, b))
5
6Output:
7a > b is True
L. ge(a, b) The ge() function returns True if a is greater than or equal to b, otherwise, it returns False.
1a = 10
2b = 10
3
4print("a >= b is", ge(a, b))
5
6Output:
7a >= b is True
M. ne(a, b) The ne() function returns True if a is not equal to b, otherwise, it returns False.
1a = 5
2b = 10
3
4print("a != b is", ne(a, b))
5
6Output:
7a != b is True
Conclusion
In this blog post, we discussed operator functions in Python. Operator functions are built-in functions that perform mathematical operations on two or more operands. We went through a list of common operator functions, such as add(), sub(), mul(), and div(). We also provided example code snippets for each operator function, along with explanations of their inputs, outputs, and use cases.
Operator functions are a useful and powerful feature of the Python programming language. They allow developers to perform complex mathematical operations with ease and simplicity. By using operator functions, developers can write more efficient and concise code, which can lead to faster execution times and better overall performance.
Looking to Learn Python? Book a Free Trial Lesson and match with top Python Tutors for concepts, projects and assignment help on Wiingy today!
FAQs
What are operators function in Python?
Operator functions in Python are pre-defined functions that allow us to perform various operations on values or variables using operators. Operators are the symbols or special characters used to perform operations on operands. In Python, operators can be used to perform arithmetic, logical, and comparison operations. Some examples of operator functions in Python are ‘+’ for addition, ‘-‘ for subtraction, ‘*’ for multiplication, and ‘/’ for division. Operator functions in Python provide a convenient and efficient way to perform operations on variables or values.
What are the 7 operators in Python?
Python has seven different types of operators, namely arithmetic, bitwise, comparison, logical, identity, membership, and assignment operators. Arithmetic operators are used to perform basic mathematical operations such as addition, subtraction, multiplication, and division. Bitwise operators perform bitwise operations on binary numbers. Comparison operators are used to compare two values and return a Boolean value. Logical operators are used to perform logical operations such as AND, OR, and NOT. Identity operators are used to compare the memory location of two objects. Membership operators are used to check whether a value is a member of a sequence or not. Assignment operators are used to assign values to variables.
What are operator functions?
Operator functions in Python are built-in functions that allow us to perform various operations on values or variables using operators. These functions are pre-defined in Python and allow us to perform arithmetic, logical, and comparison operations. For instance, the ‘+’ operator function adds two values, the ‘==’ operator function checks if two values are equal, and the ‘not’ operator function performs logical negation on a value. Operator functions provide a concise and efficient way to perform operations on variables or values in Python.
What are the 4 operators in Python?
Python has more than four operators, but if we are considering only the basic arithmetic operators, there are four: addition, subtraction, multiplication, and division. The ‘+’ operator adds two values, the ‘-‘ operator subtracts one value from another, the ‘*’ operator multiplies two values, and the ‘/’ operator divides one value by another. These operators can be used to perform basic arithmetic operations on numeric values in Python. Additionally, Python also has other types of operators such as logical, bitwise, comparison, identity, membership, and assignment operators.
Other Useful Reads
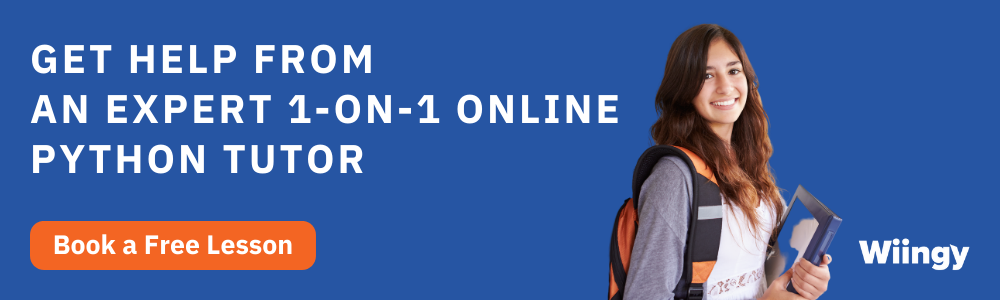
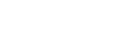
Jan 30, 2025
Was this helpful?