C++ Standard Template Library (STL) : Advanced C++ Concepts
By Rahul Lath on Jul 10, 2023
Updated Sep 27, 2024

The C++ Standard Template Library (STL) is a powerful library that forms an integral part of the C++ standard library. It provides a collection of generic classes and functions that serve as essential tools for a wide range of programming tasks. The C++ STL is designed to facilitate code reuse, improve code efficiency, and promote a more modular approach to programming.
At its core, the C++ STL embodies the principles of generic programming. It offers a set of generic algorithms, containers, iterators, and function objects, allowing developers to write code that operates seamlessly on different data types. This generic nature of the STL enables the creation of highly flexible and efficient code, as it decouples algorithms from the specific data structures they operate on.
The C++ STL consists of various components that work together harmoniously. The algorithms component provides a rich collection of functions for sorting, searching, transforming, and modifying data. The containers component offers a range of generic container classes such as vectors, lists, queues, stacks, sets, and maps, allowing efficient storage and manipulation of data. Iterators act as a bridge between algorithms and containers, providing a uniform interface for accessing and iterating over elements. Functors, or function objects, enhance the flexibility of the C++ STL by encapsulating specific behaviors that can be passed to algorithms.
By utilizing the C++ STL, developers can leverage the power of pre-implemented, highly optimized algorithms and containers, saving significant development time. The C++ STL’s emphasis on code reuse, efficiency, and flexibility has made it an indispensable tool for C++ programmers. With extensive community support and wide adoption, the C++ STL continues to be a cornerstone of modern C++ development, enabling programmers to write elegant, efficient, and maintainable code.
Looking to learn C++ programming? Book a free lesson for Online C++ Tutor and get help from Expert C++ Programmers and Software Engineers.
What is Generic Programming?
Generic programming is a programming paradigm that emphasizes writing code in a way that is independent of specific data types. It allows you to write reusable code that works with different data types, enabling you to create highly flexible and efficient algorithms. The idea behind generic programming is to separate algorithms from the data structures they operate on, resulting in more reusable and modular code.
C++ Standard Template Libbrary (STL)
The C++ Standard Template Library (STL) is a collection of generic classes and functions that provides powerful tools for various common programming tasks. It is an integral part of the C++ standard template library and has been designed to facilitate code reuse, improve code efficiency, and promote a more modular approach to programming.
What are the Components of STL?
The C++ Standard Template Library (STL) is a powerful library that provides a collection of generic classes and functions for various common programming tasks. It is a part of the C++ standard library and includes components like algorithms, containers, functors, and iterators. Let’s briefly discuss each component:
Algorithms
The algorithms component of the C++ STL consists of a set of generic algorithms that operate on containers or other sequences of data. These algorithms include operations like sorting, searching, modifying, and transforming data. They are designed to work with various container types and provide efficient and reusable solutions for common tasks.
1#include <algorithm>
2#include <vector>
3#include <iostream>
4
5
6int main() {
7std::vector<int> numbers = {5, 2, 8, 1, 9};
8
9
10
11
12// Sorting the vector
13std::sort(numbers.begin(), numbers.end());
14
15
16// Output: 1 2 5 8 9
17for (int num : numbers) {
18 std::cout << num << " ";
19}
20
21
22return 0;
23}
Containers
Containers in the C++ STL are generic classes that provide different ways to store and manage collections of objects. There are several container classes available, such as vectors, lists, queues, stacks, sets, maps, and more. Each container has its own characteristics and usage scenarios, allowing you to choose the most appropriate one for your needs.
1#include <vector>
2#include <iostream>
3
4
5int main() {
6std::vector<int> numbers = {1, 2, 3, 4, 5};
7
8
9// Adding an element to the vector
10numbers.push_back(6);
11
12
13// Output: 1 2 3 4 5 6
14for (int num : numbers) {
15 std::cout << num << " ";
16}
17
18
19return 0;
20}
Functors
Functors, also known as function objects, are objects that behave like functions. They are used in conjunction with algorithms to provide customized behavior. Functors can be created as standalone classes or implemented as lambda expressions. They are highly flexible and allow you to encapsulate specific behavior that can be passed to algorithms.
1#include <algorithm>
2#include <vector>
3#include <iostream>
4
5
6// Functor to check if a number is even
7struct IsEven {
8bool operator()(int num) const {
9return num % 2 == 0;
10}
11};
12
13
14int main() {
15std::vector<int> numbers = {1, 2, 3, 4, 5};
16
17
18// Counting even numbers using a functor
19int count
Iterators
Iterators are used to traverse and access elements within containers or other data structures. They provide a uniform interface for iterating over the elements of a container, regardless of its specific implementation. Iterators can be used in combination with algorithms to perform various operations on the underlying data.
1#include <iostream>
2#include <vector>
3
4
5int main() {
6 std::vector<int> numbers = {1, 2, 3, 4, 5};
7
8
9 // Using iterators to access and print elements
10 for (auto it = numbers.begin(); it != numbers.end(); ++it) {
11 std::cout << *it << " ";
12 }
13
14
15 return 0;
16}
Conclusion
The C++ Standard Template Library (STL) is a powerful and essential component of the C++ standard library. It provides a collection of generic classes and functions that enable programmers to write efficient and reusable code for common programming tasks. The STL consists of several components, including algorithms, containers, iterators, functors, and more.
The algorithms component of the C++ STL offers a wide range of generic functions that operate on containers or other data sequences. These algorithms provide solutions for sorting, searching, transforming, and modifying data, promoting code reuse and efficiency. The containers in the C++ STL offer various generic classes such as vectors, lists, queues, and sets, which allow for efficient storage and manipulation of data.
Iterators play a vital role in the C++ STL by providing a uniform interface to traverse and access elements within containers. They enable seamless iteration and facilitate interactions between algorithms and containers. Functors, also known as function objects, enhance the flexibility of the STL by encapsulating specific behaviors that can be passed to algorithms.
The C++ STL’s generic design and extensive functionality make it a fundamental tool for C++ programmers. Its components work together seamlessly, allowing developers to write concise and efficient code. By leveraging the C++ STL, programmers can achieve code reuse, modularity, and improved productivity. The C++ STL’s popularity and wide adoption demonstrate its significance in the C++ programming community, making it an indispensable part of modern C++ development.
Looking to learn C++ programming? Book a free lesson for Online C++ Tutor and get help from Expert C++ Programmers and Software Engineers.
FAQs
What are templates used for in C++?
Templates in C++ are used to create generic code that can work with different data types. They allow you to define generic types, functions, and classes, where one implementation can be used for multiple data types. Templates enable code reusability and help in writing flexible and efficient code by avoiding code duplication.
What is a class template and function template in C++?
In C++, a class template is a blueprint for creating classes that can work with different data types. It allows you to define a class with generic members, such as data members and member functions, that can operate on any type specified when the class is instantiated. Class templates are defined using the template keyword followed by the template parameter(s) and the class definition.
Similarly, a function template in C++ allows you to define a generic function that can operate on multiple data types. Function templates are defined using the template keyword followed by the template parameter(s) and the function definition. The function template can be instantiated with different types when it is called, allowing for code reuse.
What are the benefits of STL (Standard Template Library)?
The STL offers several benefits to C++ programmers:
Reusability: The STL provides a collection of generic algorithms, containers, and other components that can be readily used across different projects. It promotes code reuse, reducing development time and effort.
Efficiency: The algorithms and containers in the STL are designed to be efficient and optimized. They provide high-performance implementations for common operations like sorting, searching, and data manipulation.
Standardization: The STL is part of the C++ standard library, ensuring its availability and compatibility across different compilers and platforms. It provides a consistent and standardized set of tools for C++ development.
Flexibility: The STL components, such as algorithms, containers, and iterators, are highly flexible and can work with various data types. They allow for generic programming, enabling developers to write code that is independent of specific data types.
Community Support: The STL is widely adopted and has a large community of C++ developers. This means there is abundant documentation, tutorials, and resources available to help programmers learn and utilize the STL effectively.
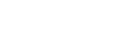
Sep 27, 2024
Was this helpful?