C++ Exceptions and Errors Handling with Examples
By Rahul Lath on Jul 24, 2023
Updated Nov 19, 2024

C++ is a powerful programming language that is widely used in software development. One of the important features of C++ is that it supports object-oriented programming (OOPs)
(Note: Object-oriented programming (OOP) is a computer programming model that organizes software design around data, or objects, rather than functions and logic like in the case of Functional Programming ).
This provides a powerful mechanism for creating complex and reusable software systems. One important aspect of OOP in C++ is the use of exceptions for gracefully handling errors and exceptions and can be user defined or built in exceptions.
Exceptions provide a powerful and rigid mechanism for handling errors and other exceptional events in a program, making it a great tool to developers . Instead of checking for errors at every step in the code, exceptions can be used to handle errors in a centralized manner at the ease of users.
Looking to learn C++ programming? Book a free lesson for Online C++ Tutor and get help from Expert C++ Programmers and Software Engineers.
C++ exception handling makes code more readable and easier to maintain. It can also be used to provide a way for functions to report errors to their callers, without requiring them to return error codes or use other error-reporting mechanisms.
In C++ exception handling uses the try-catch block, which we are going to further see in this article. The try block contains the code that may throw an exception. If an exception is thrown, control is transferred to the catch block, which handles the exception. C++ exceptions also provide several standards that can be used to handle different types of errors. Now let’s look at how we implement these with the course of this article.
Why use exceptions?
Let us discuss what is the need for C++ exceptions before we get into how to use them.
Exceptions can be used to handle various errors and other exceptional events that may occur in a program. Instead of checking for errors at every step in the code which can be very tedious, exceptions can help in handling errors in a more streamlined manner which makes the code more maintainable and easy to read.
Struggling to debug complex code issues? Learn effective strategies to identify and resolve coding errors in our blog: Debugging 101.
Exceptions can also be used to provide a way for functions to report errors to their callers (calling functions), without requiring them to return error codes or use other error-reporting mechanisms like error codes and error flags, they have their own limitation but they are whole new topics in themselves and we won’t be diving into them as of this article.
How to use exceptions?
C++ exception handling is implemented using the try-catch block.
For students tackling complex concepts like C++ exception handling in their college assignments, getting the right help is crucial. To learn more about how online tutoring can support your learning, especially with intricate topics such as these, consider reading our article on How Online Tutoring Can Help with College-Level C++ Assignments.
- The try block contains the code that may throw an exception.
- If an exception is thrown, control is transferred to the catch block, which handles the exception.
Try Exceptions:
The try block is used to enclose the code that may throw an exception. If an exception is thrown, control is transferred to the catch block.
1try {
2 // code that may throw an exception
3}
Catching Exceptions:
In C++ exception handling, the catch block is used to handle exceptions that are thrown during program execution. The catch block specifies the type of exception to catch and the actions to be taken when the exception is caught. Multiple catch blocks can be used to handle different types of exceptions. When an exception is caught, the catch block can take appropriate actions, such as logging the error, displaying an error message, or attempting to recover from the error. By using catch blocks, developers can ensure that errors are handled in a controlled and consistent manner, improving the overall reliability and maintainability of their code.
1try {
2 // code that may throw an exception
3}
4catch (exception_type1 e1) {
5 // handle exception_type1
6}
7catch (exception_type2 e2) {
8 // handle exception_type2
9}
In the above example code the catch blocks specify the type of exception they catch using the exception_type parameter. If an exception of type exception_type1 is thrown, the code in the corresponding catch block will execute to handle the exception. Similarly, if an exception of type exception_type2 is thrown, the code in the corresponding catch block will execute.
Examples of Try and Catch Exceptions
Here’s an example of a try-catch block that handles an exception that occurs when dividing by zero:
1try {
2 int x = 10;
3 int y = 0;
4 int z = x / y;
5}
6catch (const std::exception& e) {
7 std::cerr << "Exception caught: " << e.what() << std::endl;
8}
Throwing Exceptions
In C++ exception handling, the throw keyword is used to throw an exception when an error or exceptional event occurs during program execution. When an exception is thrown, control is transferred to the nearest catch block that can handle the exception. The C++ throw exception statement can be used to throw any type of exception, including built-in exceptions or user-defined exceptions. By throwing exceptions, developers can ensure that errors are handled in a centralized and consistent manner, improving the overall robustness and maintainability of their code.
1throw exception_type();
Throwing Exceptions in C++ Example
Here’s an example of a function that throws an exception if a parameter is negative:
In this code we can see that when x passed to the function “myFunction” and if it is negative then it will throw an exception displaying the error message “Parameter must be non-negative”
1void myFunction(int x) {
2 if (x < 0) {
3 throw std::invalid_argument("Parameter must be non-negative");
4 }
5 // rest of the function
6}
C++ Standard Exceptions
C++ provides several standard exceptions that can be used to handle different types of errors. These exceptions are defined in the <stdexcept> header file. Some examples include:
- std::runtime_error: This exception is used to signal runtime errors that cannot be detected at compile time.
- std::logic_error: This exception is used to signal errors in program logic.
- std::out_of_range: This exception is thrown when an index is out of range.
1try {
2 std::vector<int> v = {1, 2, 3};
3 int x = v.at(10);
4}
5catch (const std::out_of_range& e) {
6 std::cerr << "Out of range exception caught: " << e.what() << std::endl;
7}
Looking to learn C++ programming? Book a free lesson for Online C++ Tutor and get help from Expert C++ Programmers and Software Engineers.
FAQS
What is the difference between error handling and exception handling in C++?
C++ error handling involves checking for errors and taking appropriate action in code, such as returning an error code or terminating the program.
Exception handling, on the other hand, involves handling errors and other exceptional events in a streamlined manner using the try-catch block
What does it mean that exceptions separate the “good path” from the “bad path”?
Exceptions provide a way to separate the normal flow of the program from the exceptional flow. When an exception is thrown, control is transferred to the catch block, which handles the exception. This allows the program to continue executing on the “good path” instead of terminating or returning an error code
What are some ways try / catch / throw can improve software quality?
Try/catch/throw can improve software quality by streamlining c++ error handling and making the code more maintainable. These statements can also help reduce bugs by catching errors that may otherwise go unnoticed by developers. Additionally, exceptions can provide a way to report errors to the caller of a function without requiring the use of error codes or error flags
What is the difference between an error and an exception?
An error is a deviation from the normal flow of a program that can be anticipated and handled by the program.
An exception, on the other hand, is an event that occurs during the execution of a program that cannot be handled by the program at that point. C++ Exceptions provide a way to handle these exceptional events and separate the normal flow of the program from the exceptional flow
Can I throw an exception from a constructor/destructor?
Yes, you can throw an exception from a constructor or destructor. You must keep in mind that if an exception is thrown from a constructor, the object may not be fully constructed, and if an exception is thrown from a destructor, the object may not be fully destroyed.Either of the 2 could lead to memory leaks which we should handle
What should I throw?
You should throw an exception that is appropriate for the situation, such as std::runtime_error for runtime errors or std::invalid_argument for invalid arguments, these are some of the common exceptions in C++ exception handling.. It is also a good practice to create custom exception classes for specific types of errors
What should I catch?
You should catch exceptions only for those who you are prepared to handle. This may include catching specific exceptions, catching all exceptions using a catch-all handler, or using multiple catch blocks to handle different types of exceptions. It is important to handle exceptions appropriately to ensure that the program continues to execute and does not crash or terminate unexpectedly.
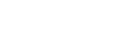
Nov 19, 2024
Was this helpful?