C++ Variables and Types: Int, Char, Float, Double, String and Bool
By Rahul Lath on Jul 10, 2023
Updated Sep 27, 2024

C++ variables are an essential part of C++ programming. In computer programming, a variable is a named location in memory that can hold a value of a certain data type. Variables in C++ programming are used to store data that can be accessed and manipulated throughout the program.
C++ programming variables are declared with a specific data type, such as integer, float, double, character, or boolean. Each C++ variable type has a specific range of values that it can hold. For example, an integer variable can hold whole numbers within a specific range (example 1-20) while a float or double variable can hold decimal values like
Variables in C++ programming can be initialized at the time of declaration or later in the program. When a variable is initialized, it is given an initial value. This value can be changed later in the program.
C++ variables can also be declared with modifiers such as const and volatile. A const variable is one whose value cannot be changed once it has been initialized. A volatile variable is one whose value can change at any time, even if the program does not modify it.
Looking to learn C++ programming? Book a free lesson for Online C++ Tutor and get help from Expert C++ Programmers and Software Engineers.
What are C++ Variables?
C++ variables are named storage locations in memory that can hold a value of a specific data type. In this article, we will explore the different types of variables in C++ and their usage.
Types of Variables?
Various C++ variable types can be used according to the needs of the programmer for their ease and need of the code.
Let us look at the different types:
Int
The int data type is used to store integer values as the name suggests. The range of values that can be stored in an int variable depends on the size of the int data type in the system being used. Let’s see an example of declaring and initializing an int variable:
1int myInt = 42;
Double
The double data type is used to store floating-point values with more precision than float. Here’s an example of declaring and initializing a double variable, we can use double to declare variables like pi, Planck’s constant, Avogadro’s number, etc
1double myDouble = 3.14159;
Char
The char data type is used to store single characters, such as letters or symbols. We can use char to store letters [‘A’ – ‘Z’], numbers [‘0’-’9’], or symbols like ‘@’,’&’,’!’ etc. They have to be initialized in quotes as we can see below. Here’s an example of declaring and initializing a char variable:
1char myChar = 'A';
Float
The float data type is used to store floating-point values with less precision than double. As we can see we have stored the value of ‘Pi’ but it is stored to only the 2nd decimal value thus showing that it stores less precision than double. Here’s an example of declaring and initializing a float variable:
1float myFloat = 3.14;
String
The string data type is used to store a sequence of characters. These characters can be letters [‘A’ – ‘Z’], numbers [‘0’-’9’], or symbols like ‘@’,’&’,’!’ etc. In C++, string literals are enclosed in double-quotes. Here’s an example of declaring and initializing a string variable:
1string myString = "Hello, World!";
Bool
The bool data type is used to store boolean values, which can be either true or false. We can even initialize them as 0 or 1 instead of true or false. Here’s an example of declaring and initializing a bool variable:
1bool myBool = true;
How to declare C++ variables?
Declaring and initializing C++ variables allows us to reserve memory for storing data of different types and assign initial values to these variables. This is an essential concept in programming as it enables us to manipulate and work with data in our programs.
By properly declaring and initializing variables, we can improve the efficiency and readability of our code, making it easier to debug and maintain.
To declare C++ variables, you need to specify their data type and name. The syntax for declaring a variable is:
1data_type variable_name;
Here is the example syntax for the cases
- I- Simply declaring a variable
- II- Initialising the variable with the number 42
- III- Declaring the variables of int type
1int myNumber; // example
2int myNumber = 42; // this also initializes the variable
3int x, y, z; // declaring multiple variables
Rules for declaring variables?
In C++ variables should have names that must adhere to a set of naming conventions and rules.
- Variable names can consist of letters, digits, and underscores
- They cannot begin with a digit.
- Additionally, variable names should be descriptive and meaningful, and they should follow a consistent naming convention, such as camelCase or snake_case.
1int age; // variable declaration using camelCase naming convention
2float hourly_rate; // variable declaration using snake_case naming convention
Variable Name or Identifiers
In C++, a variable name or identifier is a user-defined name that is used to identify a variable in a program. Variable names must follow certain rules
- Starting with a letter or underscore
- Not containing spaces
- Not being a reserved keyword
Here is an example of declaring and using a variable named myVar:
1int myVar = 42; // declaring and initializing a variable
2cout << "The value of myVar is " << myVar << end; // using the variable
Defining Constants with the ‘const’ Keyword
In C++, constants can be defined using the const keyword. Constants are variables whose values cannot be changed once they are defined. Defining constants can help make code more readable and prevent accidental modifications of important values.
1const float PI = 3.14159;
2const int MAX_NUM = 100;
Scope of Variables in C++
The scope of a variable in C++ refers to the region of the program where the variable is visible and can be accessed. There are three types of variable scope: global, local, and class scope. Global variables are visible throughout the entire program, local variables are visible only within the block in which they are declared, and class variables are visible within the class in which they are declared. Here is an example of declaring and using a local variable named myVar:
1void myFunction()
2{
3 int myVar = 42; // declaring a local variable
4 cout << "The value of myVar is " << myVar << endl; // using the variable
5}
6
7
8int main()
9{
10 myFunction();
11 return 0;
12}
Variable Type Conversion
Variable type conversion in C++ refers to the process of converting one data type to another. This can happen implicitly, where the compiler automatically converts the data type, or explicitly, where the programmer specifies the conversion using casting. Here is an example of implicit conversion from int to float:
1int num = 10;
2float result = num / 2; // implicit conversion from int to float
3cout << "The result is " << result << endl;
Register Variables
A register variable in C++ is a type of variable that is stored in a CPU register instead of in memory. Register variables can be accessed faster than normal variables, but they are limited in number and size. Here is an example of declaring a register variable:
1register int num = 10; // declaring a register variable
Escape Sequences
Escape sequences in C++ are special characters that are used to represent non-printable characters or to produce special effects in output. Some common escape sequences include \n for a newline character, \t for a tab character, and \” for a double quote character. Here is an example of using escape sequences in a string:
1cout << "Hello\tworld!\n" << end; // using escape sequences in a string
Looking to learn C++ programming? Book a free lesson for Online C++ Tutor and get help from Expert C++ Programmers and Software Engineers.
FAQS
What is the Difference Between Variable Declaration and Definition?
Variable declaration is the process of telling the compiler the data type and name of a variable, while the variable definition is the process of allocating memory for the variable and assigning it an initial value.
What are the 7 basic data types in C++?
The 7 basic data types in C++ are bool, char, int, float, double, void, and wchar_t.
What is the variable in OOP?
In OOP, a variable (also known as an instance variable or a member variable) is a member of a class that can hold a value. Each instance of the class has a separate copy of the variable.
References
- The C++ Programming Language (4th Edition) By Bjarne Stroustrup: https://www.stroustrup.com/4th.html
- C++ Pocket Reference 1st Edition Accelerated C++: https://www.oreilly.com/library/view/c-pocket-reference/9780596801762/
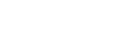
Sep 27, 2024
Was this helpful?