Diving Deeper: Understanding Functions and Arrays in C++
By Rahul Lath on Jul 10, 2023
Updated Sep 27, 2024

In this article we delve into an essential component in c++: Functions. Functions are an essential part of C++ programming.
They allow you to break down your code into smaller, more manageable pieces that can be reused throughout your program. In C++ functions are blocks of code that perform a specific task.
You can think of them as mini-programs within your main program. Functions can take input, perform operations on that input, and return output.
Looking to learn C++ programming? Book a free lesson for Online C++ Tutor and get help from Expert C++ Programmers and Software Engineers.
There are several types of C++ functions, including void functions, value-returning functions, and reference-returning functions.
- Void functions do not return a value and are used when you need to perform a task without returning a result.
- Value-returning functions return a value of a specific data type, such as an integer or a string.
- Reference-returning functions return a reference to a variable, which allows you to modify the original variable’s value.
Let’s take a look at an example of C++ functions. Suppose you want to write a program that calculates the area of a circle. You could write a function that takes the radius of the circle as input, performs the necessary calculations, and returns the area of the circle. This function would be a value-returning function, as it returns a value (the area of the circle) of type float or double.
In summary, C++ functions are an essential tool for organizing and reusing code. They come in several types, including void functions, value-returning functions, and reference-returning functions. By writing functions, you can make your code more modular and easier to understand.
Syntax
1return_type function_name (parameter1, parameter2, ...) {
2 // code to be executed
3 return value;
4}
- return_type is the type of value that the function returns.
- function_name is the name of the function
- parameters are the input values that the function takes
- code block inside the function is executed when the function is called
- return statement specifies the value that the function returns.
Here’s an example of a function that takes two integers as input and returns their sum:
1int sum(int a, int b) {
2 int result = a + b;
3 return result;
4}
In this example, the function is named “sum”, and it takes two integers ‘a’ and ‘b’ as input, you can use any variable name . The function then adds the variables and returns the result.
Why do we need functions?
In C++ functions are vital for many reasons.
- They allow us to modularize code and make it easier to read and understand.
- They also allow us to reuse code, which saves time and reduces errors.
- They make the code more efficient by breaking it down into smaller, more manageable parts.
Types of functions
There are two types of function in c++ types: standard library functions and user-defined functions. Standard library functions are built-in functions that are provided by the C++ standard library, such as “printf” and “sqrt”. User-defined functions are functions that are created by the programmer.
C++ Function Declaration
C++ function declaration is a way to tell the compiler that a function of this name exists. This is done by specifying the function name, return type, and parameter types. Here’s the syntax of a function declaration:
1return_type function_name(parameter_list);
- return_type is the data type of the value that the function returns
- function_name is the name of the function
- parameter_list is the list of input parameters the function takes.
Here is the function in c++ example declaration:
1int sum(int a, int b);
In this example, the sum function is declared with a return type of int and two integer parameters a and b
Calling a Function
Once a function is declared, you can call it from anywhere in the program.
Syntax of calling in C++ functions:
1function_name(argument_list);
- function_name is the name of the function
- argument_list is the list of values passed to the function as input parameters.
Calling a function in c++ example :
1int result = sum(5, 10);
In this example, the sum function is called with two integer arguments, and the returned value is assigned to the variable result.
Parameter Passing to Functions
In C++ functions, there are two ways to pass arguments to a function:
- call by value
- call by reference.
In call by value, the value of the argument is passed to the function. Any changes made to the argument inside the function do not affect the original value of the argument.
Call by value function in c++ example
1void increment(int x) {
2 x++;
3}
4int main() {
5 int a = 10;
6 increment(a);
7 cout << a; // Output: 10
8}
In call by reference, the memory address of the argument is passed to the function. Changes made to the argument inside the function affect the original value of the argument.
Call by reference function in c++ example:
1void increment(int& x) {
2 x++;
3}
4int main() {
5 int a = 10;
6 increment(a);
7 cout << a; // Output: 11
8}
Functions using Pointers
Functions using pointers in C++ are a powerful tool for passing variables by reference to a function. A pointer is a variable that stores the memory address of another variable. Pointers can be used to manipulate variables indirectly and can be used to pass a variable by reference to a function.
Pointers in function in c++ example:
1void swap(int* x, int* y) {
2 int temp = *x;
3 *x = *y;
4 *y = temp;
5}
6int main() {
7 int a = 10, b = 20;
8 cout << "Before swap: a = " << a << ", b = " << b << endl;
9 swap(&a, &b);
10 cout << "After swap: a = " << a << ", b = " << b << endl;
11}
In this example, the swap function takes two integer pointers as input parameters. The function body swaps the values of the two variables pointed to by the pointers. The main function calls the swap function by passing the addresses of the variables a and b using the & operator.
Points to remember about functions in C++
- C++ functions can have multiple parameters of different data types.
- C++ functions can have a return type of void, which means it does not return any value.
- C++ functions can have a default argument, which is used when no argument is provided.
- C++ functions can be overloaded, which means multiple functions can have the same name but different parameter lists.
- C++ functions can be declared as static or extern, which affects its scope and linkage.
- C++ functions can call itself recursively, which is called recursion.
(Function Overloading,Storage classes and Recursion will be covered in the later section)
C++ Function Overloading
C++ function overloading allows multiple functions with the same name but different parameter lists to coexist in the same program. A confusion that arises while programming this is that if the return type has to be the same, the answer to which is- No, it is not necessary for the functions with the same name to have same return type. This is useful when you want to perform the same operation on different data types(int, float,string etc)
1int sum(int a, int b) {
2 return a + b;
3}
4float sum(float a, float b) {
5 return a + b;
6}
7int main() {
8 cout << sum(2, 3) << endl; // Output: 5
9 cout << sum(2.5f, 3.5f) << endl; // Output: 6
10}
In this example, there are two sum functions with the same name but different parameter lists. The first sum function takes two integer parameters and returns their sum, while the second sum function takes two float parameters and returns their sum.
C++ Default Argument
C++ default arguments are used to provide a default value for a function parameter if no argument is provided when the function is called.
1int power(int x, int y = 2) {
2 int result = 1;
3 for (int i = 0; i < y; i++) {
4 result *= x;
5 }
6 return result;
7}
8int main() {
9 cout << power(3) << endl; // Output: 9
10 cout << power(3, 3) << endl; // Output: 27
11}
In this example, the power function takes two integer parameters, x and y. The y parameter has a default value of 2. If no value is provided for y when the power function is called, it will use the default value of 2.
Static variable function in c++ example:
1void counter() {
2 static int count = 0;
3 count++;
4 cout << "Count: " << count << endl;
5}
6int main() {
7 counter(); // Output: Count: 1
8 counter(); // Output: Count: 2
9 counter(); // Output: Count: 3
10}
In this example, the counter function uses a static variable, count, which is initialized to 0. The count variable is incremented each time the counter function is called, and the value of count is printed to the console.
C++ recursion is a technique in which a function calls itself. Recursion is used to solve problems that can be broken down into smaller subproblems.
1int factorial(int n) {
2 if (n == 0) {
3 return 1;
4 } else {
5 return n * factorial(n - 1);
6 }
7}
8int main() {
9 cout << factorial(5) << endl; // Output: 120
10}
In this example, the factorial function is defined recursively. The function takes an integer parameter, n, and returns the factorial ofn. The base case for the recursion is when n is 0, in which case the function returns 1. Otherwise, the function multiplies n by the result of calling factorial with n-1.
C++ passing arrays to a function allows a function to operate on an array without needing to know the array’s size or contents.
1void printArray(int arr[], int size) {
2 for (int i = 0; i < size; i++) {
3 cout << arr[i] << " ";
4 }
5 cout << endl;
6}
7int main() {
8 int arr[] = {1, 2, 3, 4, 5};
9 int size = sizeof(arr) / sizeof(arr[0]);
10 printArray(arr, size); // Output: 1 2 3 4 5
11}
In this example, the printArray function takes an integer array and its size as input parameters. The function loops through the array and prints each element to the console. The main function defines an integer array and its size, and then calls the printArray function with the array and its size.
The return statement in C++ is used to return a value from a function. The return type of a function must match the data type of the value being returned.
1int add(int a, int b) {
2 return a + b;
3}
4int main() {
5 int result = add(2, 3);
6 cout << result << endl; // Output: 5
7}
In this example, the add function takes two integer parameters and returns their sum using the return statement. The main function calls the add function and assigns the returned value to the variable result, which is then printed to the console.
C++ Storage Class
In C++ programming, a storage class is used to specify the scope and lifetime of a variable. There are four types of storage classes in C++:
- Auto
- Register,
- Static
- extern.
- auto storage class::It is used by default in C++ programming. Variables declared with the auto storage class are local to the block in which they are declared, and their lifetime is the block they are declared in.
- register storage class:: It is used to define local variables that should be stored in a register instead of memory. This can result in faster access to the variable.
- static storage class:: It is used to define variables that retain their values between function calls. Static variables have a lifetime that extends beyond the block in which they are declared.
- extern storage class:: It is used to declare variables that are defined in another file. Extern variables are global in scope, and their lifetime is the entire program.
1#include <iostream>
2using namespace std;
3
4
5void increment() {
6 static int count = 0; // static variable
7 count++;
8 cout << "Count is " << count << endl;
9}
10
11
12int main() {
13 increment();
14 increment();
15 increment();
16 return 0;
17}
The output is:
1Count is 1
2Count is 2
3Count is 3
C++ Recursion
Recursion is a technique in which a function calls itself. It can be useful for solving problems that can be broken down into smaller, similar sub-problems. Recursive functions have a base case to help in terminating the function so it doesnt run into an infinite loop and give a run time error.
1#include <iostream>
2using namespace std;
3
4
5int factorial(int n) {
6 if (n == 0) {
7 return 1; // base case
8 } else {
9 return n * factorial(n-1); // recursive case
10 }
11}
12
13
14int main() {
15 int n = 5;
16 cout << "Factorial of " << n << " is " << factorial(n) << endl;
17 return 0;
18}
Output is:
1Factorial of 5 is 120
C++ Passing Array to Function
In C++ programming, arrays are passed to functions by reference. This means that any changes made to the array inside the function will affect the original array outside the function.
(Remember call by reference we discussed before)
1#include <iostream>
2using namespace std;
3
4
5void printTheArray(int arr[], int size) {
6 for (int i = 0; i < size; i++) {
7 cout << arr[i] << " ";
8 }
9 cout << endl;
10}
11
12
13int main() {
14 int arr[] = {1, 2, 3, 4, 5};
15 int size = sizeof(arr) / sizeof(arr[0]);
16 printTheArray(arr, size);
17 return 0;
18}
Output is:
11 2 3 4 5
In this example, the printTheArray function takes an array arr and its size as the arguments of the function and prints the elements.
Return Statement
The return statement in C++ programming is used to return a value from a function. It can be used anywhere in the code to terminate the function after necessary condition is matched or necessary value is obtained. Functions can return any data type. C++ functions can only return one value, but that value can be anything like a complex structure (struct) or range from int to string.
1#include <iostream>
2using namespace std;
3
4
5int add(int a, int b) {
6 return a + b;
7}
8
9
10int main() {
11 int x = 5, y = 10;
12 int sum = add(x, y);
13 cout << "Sum is " << sum << endl;
14 return 0;
15}
Output is:
1Sum is 15
In this example, the add function takes two integers a and b as arguments and returns their sum. The main function calls add with the values of x and y and assigns the result to sum.
FAQS
What are the Benefits of Using User-Defined Functions in C++?
There are several benefits of using user-defined C++ functions. Some of them are:
Reusability: Functions can be reused in the program, reducing the amount of code.
Modularity: Functions can be used to break down a large program into smaller, more manageable parts.
Simplification:Functions help in simplifying complex tasks.
Improved readability: They can improve the readability of the code by encapsulating complex logic into a single function easily
Does C++ have a type function?
C++ does not have a built-in type function. Nevertheless, the typeid operator is often used to determine the type of a variable at runtime. Here’s an example:
1#include <iostream>#include <typeinfo>using namespace std;int main() { int x = 5; double y = 3.14; cout << "Type of x is " << typeid(x).name() << endl; cout << "Type of y is " << typeid(y).name() << endl; return 0;}
Output is:
1Type of x is iType of y is d
Can a C++ function return more than one value?
A C++ function can only return one value, but that value can be anything like a complex structure (struct) or range from int to string.
Can a C++ function call itself?
Yes, a C++ function can call itself and this is called recursion where a complex task can be recursively solved by breaking into smaller tasks.
References
- 1. The C++ Programming Language (4th Edition) By Bjarne Stroustrup: https://www.stroustrup.com/4th.html
- C++ Pocket Reference 1st Edition Accelerated C++: https://www.oreilly.com/library/view/c-pocket-reference/9780596801762/
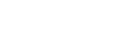
Sep 27, 2024
Was this helpful?