Proficiency in manipulating, analyzing, and visualizing data is critical in today’s technology-driven society. RStudio and its suite of packages, specifically the Tidyverse in RStudio, are instrumental in this regard.
To clarify, the Tidyverse comprises a compilation of R packages that have been specifically developed for the purpose of data science. By virtue of their shared APIs and underlying philosophy, all packages comprising the Tidyverse simplify and streamline data analysis in R.
Whether you are an experienced professional or a novice data scientist, gaining knowledge of the Tidyverse will greatly improve your proficiency in R programming. This tutorial will introduce you to Tidyverse by discussing its fundamental packages, advantages, and the way to integrate it seamlessly into your RStudio workflow.
Looking for R Programming help? Book a free lesson with Wiingy and get matched with expert RStudio Tutors for data analysis, statistical modeling, and more.
What is Tidyverse?
The Tidyverse is not just a single package but a collection of multiple packages that provide a cohesive set of tools for data analysis in R. Each package specializes in a particular aspect of data analysis, ensuring that users have a comprehensive toolkit at their disposal.
Here’s a breakdown of some of the core Tidyverse packages:
ggplot2: This package is all about data visualization. Instead of thinking about plots in terms of dots and lines, ggplot2 uses consistent and intuitive grammar. For instance, to create a scatter plot, you might use:
1library(ggplot2)
2
3ggplot(data = mtcars, aes(x = wt, y = mpg)) +
4
5 geom_point()
dplyr: When it comes to data manipulation, dplyr is your go-to. It offers functions like filter(), arrange(), and mutate() that make data wrangling a breeze.
1library(dplyr)
2
3mtcars %>%
4
5 filter(mpg > 20) %>%
6
7 arrange(desc(mpg))
tidyr: This package focuses on tidying your data. With functions like spread() and gather(), you can reshape data frames with ease.
readr: Forget the base R read functions; readr provides a fast and friendly way to read rectangular data.
purrr: For functional programming in R, purrr is a game-changer. It enhances R’s functional programming toolkit.
tibble: Tibbles are a modern reimagining of data frames in R, providing a cleaner display and better subsetting.
stringr: String manipulation becomes straightforward with stringr, whether you’re extracting, detecting, or counting.
forcats: Categorical data, often overlooked, gets the attention it deserves with forcats, simplifying tasks like reordering or recoding factors.
Each of these packages, when used individually, offers a robust set of tools. But when used in tandem, they provide a cohesive and streamlined data analysis workflow in RStudio.
Setting Up Tidyverse in RStudio
Before learning about Tidyverse, we need to get it set up in RStudio. Don’t worry; the process is straightforward.
- Installing the Tidyverse package: If you’ve never installed Tidyverse before, it’s as simple as running:
1install.packages("tidyverse")
This command will install all core Tidyverse packages. The beauty is that you don’t need to install each package individually.
- Loading the Tidyverse library: Once installed, you can load the Tidyverse suite into your R session with:
1library(tidyverse)
Upon loading, you might notice a message indicating the packages that have been loaded, and those that have been masked. This is standard and provides clarity on package functions and potential overlaps.
Now that you’re set up let’s have a look at a typical Tidyverse workflow.
Tidyverse Workflow
Understanding and mastering the Tidyverse workflow can immensely elevate your data analysis efficiency. The synergy between the Tidyverse packages allows for a seamless, logical flow of data manipulation, visualization, and analysis. Here’s a glimpse into a typical workflow:
- Data Import with readr: Begin by importing your dataset. With readr, you can easily read in CSVs, TSVs, and other rectangular data formats.
1library(readr)
2
3data <- read_csv("path_to_your_file.csv")
- Data Wrangling with dplyr and tidyr: Once your data is in R, you can start manipulating it. Maybe you want to filter out certain rows, create a new variable, or reshape the data.
1library(dplyr)
2
3library(tidyr)
4
5# Filter data where 'mpg' is greater than 20 and arrange by 'hp'
6
7filtered_data <- data %>%
8
9 filter(mpg > 20) %>%
10
11 arrange(hp)
- Visualization with ggplot2: Now, let’s say you want to visualize the relationship between ‘mpg’ and ‘hp’ from the filtered_data.
1library(ggplot2)
2
3ggplot(filtered_data, aes(x = hp, y = mpg)) +
4
5 geom_point() +
6
7 labs(title = "Relationship between Horsepower and Miles per Gallon")
Functional Programming with purrr: Perhaps you’d like to apply a function across elements of a list or vector.
1library(purrr)
2
3list(1:5, 6:10) %>%
4
5 map(~.x^2)
This is just scratching the surface, but you can see how each step logically flows into the next, making the entire process intuitive and efficient.
Benefits of Using Tidyverse in RStudio
The Tidyverse isn’t just a set of packages; it’s a paradigm shift in the way we approach data analysis in R. Here’s why so many swear by it:
Improved Data Analysis Speed: The consistent and intuitive syntax across Tidyverse packages means less time looking up function arguments and more time analyzing data.
Consistency and Readability in Code: A major advantage is the clarity and consistency in code, making it easier for others (and your future self!) to understand.
Rich Ecosystem and Community Support: The active community ensures that the packages are continuously updated, and any issues or queries are addressed promptly. The vast array of online tutorials, forums, and support from expert rstudio tutors ensures you’re never left in the dark.
Integration with Advanced R Features: Whether it’s R Markdown, Shiny apps, or advanced statistical modeling, Tidyverse integrates seamlessly.
Common Challenges and Solutions
While the Tidyverse offers a streamlined approach to data analysis in R, beginners might face some hurdles. Here are common challenges and their solutions:
- Understanding the %>% (Pipe Operator):
This operator, which comes from the magrittr package, allows you to chain functions together. Think of it as “then do this.”
Solution: Practice is key. Start with simple chains and gradually increase complexity.
1mtcars %>%
2
3 filter(mpg > 20) %>%
4
5 select(mpg, hp)
- Managing Different Data Formats:
Tidyverse prefers tibbles to traditional data frames, which can sometimes cause confusion. Solution? Familiarize yourself with the as_tibble() and as.data.frame() functions to switch between formats when needed.
- Dealing with Warning Messages:
Sometimes, when loading the Tidyverse, you might encounter warning messages about masked objects.
Here is the solution- This is a normal message indicating that some functions from the Tidyverse packages might overlap with those in Base R or other packages. Always ensure you’re using the function from the intended package.
- Handling Large Datasets:
While Tidyverse functions are optimized, handling very large datasets might cause performance issues.
Solution: Consider using data.table for data manipulation or the dtplyr package, which allows you to write dplyr code that’s executed by data.table.
Advanced Tidyverse Techniques
Once you’re comfortable with the basics, you can harness even more power from the Tidyverse by learning the advanced techniques:
Leveraging map functions in purrr
The purrr package offers a suite of map functions that allow for more concise and readable iterations.
1library(purrr)
2
3list(1:3, 4:6) %>%
4
5 map(~mean(.x))
Creating Custom ggplot2 Themes
Beyond the default themes in ggplot2, you can create your own for personalized and consistent visuals.
1my_theme <- function() {
2
3 theme_minimal() +
4
5 theme(text = element_text(color = "blue"))
6
7}
8
9ggplot(mtcars, aes(mpg, hp)) +
10
11 geom_point() +
12
13 my_theme()
Efficient Data Wrangling with dplyr and tidyr
Going deep into the functions like group_by, summarise, pivot_longer, and pivot_wider gives more complex data manipulations options.
Tidyverse vs. Base R: When to Use What
While Tidyverse offers a plethora of tools and a modern approach to data analysis, it’s crucial to understand when to use it versus Base R:
Consistency vs. Simplicity
Tidyverse offers consistent syntax, making it easier to remember and chain functions. However, for simple, one-off tasks, Base R might be quicker.
Performance
For very large datasets, some Base R functions or other packages like data.table might offer better performance.
Learning Curve
Beginners often find the Tidyverse syntax more intuitive and readable, making it a popular choice for teaching.
Functionality
Some advanced statistical functions are not available in the Tidyverse and require Base R or other specialized packages.
Learning data science and analysis its with RStudio becomes significantly more efficient and intuitive with the rstudio tidyverse suite. It offers a cohesive set of tools that synergize well, ensuring that you can handle everything from data wrangling to visualization with ease. While the Tidyverse might seem overwhelming initially, with practice and the resources at hand, it quickly becomes second nature.
And remember, every tool has its place. While the Tidyverse is powerful and modern, Base R and other packages still hold their merit in specific scenarios. Equip yourself with the knowledge of both, and you’ll be well-prepared for any data challenge thrown your way. Should you ever need personalized guidance, remember that expert Rstudio tutors are always available to assist.
Looking for R Programming help? Book a free lesson with Wiingy and get matched with expert RStudio Tutors for data analysis, statistical modeling, and more.
FAQs
Why is there so much emphasis on the Tidyverse when R has a rich ecosystem of packages?
The Tidyverse has been designed with a consistent philosophy and shared APIs, making it easier to learn and use. The integration across packages ensures a seamless data analysis experience.
I’m comfortable with Base R. Do I need to learn the Tidyverse?
While Base R is powerful, the Tidyverse offers a more modern approach to data analysis, especially for data wrangling and visualization. Learning it can enhance your efficiency and the readability of your code.
Can I mix Tidyverse functions with Base R functions in my code?
Absolutely! You can use both Tidyverse and Base R in the same script. But keep in mind that some functions may overlap, and make sure you are using the right functions from the right packages.
Are there any performance issues with the Tidyverse when handling large datasets?
There are functions in Tidyverse that are best for general use, but some packages, like data.table, might work better with very large datasets.
I’m facing errors with a specific Tidyverse function. Where can I seek help?
The R community is big and helpful. R-specific forums can be found on sites like Stack Overflow. Additionally, you can always turn to expert rstudio tutors for in-depth guidance.
How often are Tidyverse packages updated?
The Tidyverse has a lively community that makes sure that its packages are always up to date. To get new features and bug fixes, it is a good idea to check for updates on a regular basis.
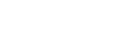
Jan 29, 2025
Was this helpful?