Web Scraping with R- Step-by-Step Guide 2025
By Rahul Lath on Nov 15, 2023
Updated Jan 29, 2025

Extensive quantities of information are readily accessible to us. However, have you ever contemplated the methodical process of extracting particular data from websites? Welcoming you to the realm of web scraping with R.
At its core, web scraping is a technique to extract information from web pages automatically. Web scraping automates the tedious and time-consuming process of manually copying data, thereby increasing its efficiency and scalability. Web scraping is a highly effective tool that can be utilized for various purposes, including field research on market trends, sentiment analysis of social media, and data collection for academic projects.
Nonetheless, great power is accompanied by great responsibility. Before delving into the world of scraping, it is vital to have a firm grasp of the ethical implications. Exceeding the permitted limits of website scraping may result in the banishment of your IP address. Thus, the robots.txt file is utilized. This file, which is situated in the root directory of a website, specifies which pages may be scraped and accessed by automated scripts. Constantly ensure that you abide by the terms of service and these guidelines of the website.
Looking for R Programming help? Book a free lesson with Wiingy and get matched with expert RStudio Tutors for data analysis, statistical modeling, and more.
Essential Tools in R for Web Scraping
R, known for its robust data analysis capabilities, also offers a suite of packages to make web scraping a breeze. Here are the essential ones:
- rvest: Inspired by Python’s Beautiful Soup, rvest is your primary weapon for web scraping. With functions like html_nodes() and html_text(), extracting data becomes straightforward.
- xml2: While rvest helps in the actual scraping, xml2 assists in parsing the fetched HTML and XML content. This package is particularly useful when dealing with more complex web structures.
- httr: Sometimes, you need more control over your HTTP requests, like adding headers or using different request methods. httr steps in here, offering functions like GET(), POST(), and more.
1library(httr)
2
3response <- GET("https://api.example.com/data")
4
5content <- content(response, "text")
Basics of Web Page Structure
Before delving into the practical aspect of web scraping, it’s crucial to understand the structure of web pages. Websites are primarily built using HTML (HyperText Markup Language). Think of HTML as the skeleton of a webpage. Elements in HTML are represented by tags, and these tags define different parts of a web page such as headings, paragraphs, links, and more.
For instance:
1<html>
2
3 <head>
4
5 <title>Sample Page</title>
6
7 </head>
8
9 <body>
10
11 <h1>Welcome to Web Scraping</h1>
12
13 <p>This is a sample paragraph.</p>
14
15 </body>
16
17</html>
Your First Web Scraping Project in R
Embarking on your first web scraping project can be both exciting and a bit daunting. But fret not! With the right guidance and tools, you’ll be a pro in no time.
Choosing a Website
First and foremost, you need a website to scrape. For beginners, I recommend choosing a website that’s:
- Static: These websites display the same content for all users, making it easier to scrape. Dynamic websites, which change content based on user interactions, are trickier and require advanced techniques.
- Legally and ethically scrapable: Always check a website’s robots.txt file by appending /robots.txt to the site’s base URL (like https://example.com/robots.txt). This file will tell you which parts of the site can be scraped.
Extracting Data with rvest
Once you’ve chosen a website, it’s time to extract data. Let’s assume you’re interested in scraping article titles from a blog.
- Inspect the Web Page: Right-click on the webpage element you’re interested in and select ‘Inspect’ or ‘Inspect Element’. This will open the browser’s developer tools, showing the HTML structure. Identify the tag and class of the data you want.
- Scrape the Data: Using rvest, fetch and parse the content.
1library(rvest)
2
3# Define the URL
4
5url <- "https://blog.example.com"
6
7# Read the webpage content
8
9webpage <- read_html(url)
10
11# Extract titles based on the identified class
12
13titles <- webpage %>% html_nodes(".article-title-class") %>% html_text()
Cleaning Data
After extraction, the data might require some cleaning. This step ensures that your data is ready for analysis or storage.
- Removing Unwanted Characters: If your scraped data has unwanted characters, you can remove them using the gsub() function.
1cleaned_titles <- gsub("\n|\r", "", titles)
2
3cleaned_titles <- gsub("\n|\r", "", titles)
- Converting Data Types: Ensure your data is in the right format. For instance, if you’ve scraped prices as character strings, convert them to numerical values using as.numeric().
Advanced Web Scraping Techniques
As you become more proficient in web scraping, you’ll encounter scenarios that require more advanced techniques.
Handling Pagination
Many websites split content across multiple pages. To scrape data from all pages, you’ll need to navigate through each one.
- Identify the Pagination Structure: Check the URL structure as you click through pages. It might look like https://example.com/page/2.
- Loop Through Pages: Use a loop to iterate through page numbers, adjusting the URL each time.
1base_url <- "https://example.com/page/"
2
3# Assuming there are 5 pages
4
5all_titles <- list()
6
7for(i in 1:5){
8
9 full_url <- paste0(base_url, i)
10
11 page_data <- read_html(full_url) %>% html_nodes(".article-title-class") %>% html_text()
12
13 all_titles <- append(all_titles, page_data)
14
15}
Dealing with JavaScript
Some websites load content using JavaScript, which rvest cannot handle directly. For such sites, tools like RSelenium or V8 can be used.
- RSelenium: This package allows R to control a web browser, mimicking human interactions. It can “see” content loaded by JavaScript.
- V8: It’s a JavaScript engine for R, enabling you to run JavaScript code directly from R.
Rate Limiting
Websites might limit the number of requests you can make in a given time frame to prevent overloading their servers. Respect these limits!
- Introduce Delays: Use the Sys.sleep() function in R to introduce pauses between requests.
- Monitor HTTP Headers: The httr package can inspect headers, which often indicate if you’re nearing a rate limit.
Storing Scraped Data
After successfully scraping your data, the next step is to store it in a structured and accessible format. Depending on the size of your data and its intended use, there are several ways to go about this:
Saving Data into CSV or Excel
CSV (Comma-Separated Values) and Excel are popular formats for storing tabular data.
To CSV: R provides the write.csv() function to store data frames into CSV format.
1# Assuming 'data' is your scraped data frame
2
3write.csv(data, file = "scraped_data.csv")
To Excel: The writexl package allows you to save data frames to Excel format without dependencies.
1library(writexl)
2
3write_xlsx(data, path = "scraped_data.xlsx")
Considerations for Large-Scale Data Scraping Projects
When dealing with massive datasets, traditional methods might not be the best fit. Instead, consider:
- Databases: R can connect to various databases, allowing you to store and retrieve data efficiently. The DBI and RSQLite packages are excellent starting points for integrating R with databases.
- Cloud Storage: Platforms like AWS S3, Google Cloud Storage, or Azure Blob offer vast storage spaces and are particularly suitable for big data projects.
Handling Common Challenges while Web Scraping in R
Web scraping isn’t always a smooth ride. You might encounter obstacles that can halt or impede your scraping endeavors. Let’s discuss some of these challenges and their solutions:
Dealing with Captchas
Captcha is a system designed to determine whether the user is human. It’s a roadblock for scrapers.
- Manual Bypass: For small projects, you might consider solving the captcha manually and then proceeding with your scraping.
- Automation Tools: Some tools and services can solve captchas automatically, though they’re not always successful, and ethical considerations arise.
Managing Changing Website Structures
Websites evolve, and their structure can change, breaking your scraping code.
- Regular Monitoring: Schedule regular runs of your scraping script to detect any failures early.
- Flexible Parsing: Instead of relying heavily on specific classes or IDs, which can change, try to find more stable elements or attributes in the web page’s structure.
Handling Errors and Exceptions
Your scraping script can sometimes fail due to unforeseen errors.
- Error Handling: Use the tryCatch() function in R to gracefully handle errors without stopping the entire script.
1result <- tryCatch({
2
3 # Your scraping code here
4
5}, warning = function(war) {
6
7 print(war)
8
9}, error = function(err) {
10
11 print(err)
12
13}, finally = {
14
15 print("Scraping attempt finished.")
16
17})
- Logging: Keep a log of your scraping activities, noting successes and failures. This way, you can revisit and debug issues.
Tips for Efficient Web Scraping in R
Web scraping is more than just extracting data—it’s about doing it efficiently, ethically, and sustainably. Here are some pro tips to enhance your web scraping journey:
Keeping Your Code Modular
One of the cornerstones of efficient coding is modularity. Breaking your scraping process into reusable functions or modules can save time and make debugging easier.
1# A function to scrape article titles
2
3scrape_titles <- function(url) {
4
5 page <- read_html(url)
6
7 titles <- page %>% html_nodes(".article-title") %>% html_text()
8
9 return(titles)
10
11}
12
13# Use the function
14
15blog_titles <- scrape_titles("https://blog.example.com")
Monitoring and Logging Your Scraping Tasks
Keep a tab on your scraping activities. Using tools like log4r can help you maintain logs, making it easier to trace back errors or changes in the website structure.
Staying Updated
Web scraping is a dynamic field. Websites change, and so do the tools we use. Regularly update your R packages and be on the lookout for changes in the website’s structure or terms of service.
Tidyverse vs. Base R: When to Use What
R is an incredibly versatile language, and when it comes to data manipulation, both Base R and the Tidyverse have their strengths.
Comparison of Functions and Capabilities
Data Manipulation: While Base R provides functions like subset(), merge(), and aggregate(), Tidyverse, through dplyr, offers a more intuitive and consistent set of verbs like filter(), select(), arrange(), and mutate().
1# Using Base R
2
3subset_data <- subset(data, condition)
4
5# Using dplyr
6
7library(dplyr)
8
9filtered_data <- data %>% filter(condition)
Data Reshaping: Base R’s reshape2 package offers melt() and dcast() for reshaping data. In contrast, Tidyverse’s tidyr provides gather() and spread() for the same.
Readability: Tidyverse, with its %>% (pipe operator), provides a more readable and chainable syntax, making the code easier to follow.
Situations Where One Might Be Preferred Over the Other
- Familiarity: If you’re more accustomed to Base R, it might be quicker for you. However, for beginners, the Tidyverse often offers a gentler learning curve.
- Performance: For specific tasks, especially on large datasets, Base R functions might be faster. However, the difference is often negligible for everyday tasks.
Web scraping with R is a powerful technique, opening doors to vast amounts of data available on the web. Whether you’re researching, building a data-driven project, or just curious, the tools in R, combined with best practices, can make your scraping endeavors fruitful. Remember always to respect website terms, stay ethical, and keep learning. The digital world is vast, and there’s always something new to discover.
Looking for R Programming help? Book a free lesson with Wiingy and get matched with expert RStudio Tutors for data analysis, statistical modeling, and more.
FAQs
Is web scraping legal?
Several factors, including the website’s terms of service, robots.txt file, and regional legislation, influence the legality of web scraping. Ensure you have the proper authorization to access and scrape the data of interest at all times.
How do I avoid getting banned while scraping?
Consider implementing the suggestions in the robots.txt file, delaying your requests, rotating user agents, and potentially utilizing proxy servers. A site’s prohibition or blocking, on the other hand, should prompt you to reevaluate your scraping strategy.
Can I scrape dynamic websites with R?
Although it is more complicated, yes. You are able to interact with web pages, including those that load content dynamically using JavaScript, using tools such as RSelenium.
Do I need to know HTML and CSS for web scraping?
A basic understanding of HTML and CSS will be beneficial, as it will allow you to identify the data you want to extract more easily.
Are there alternatives to R for web scraping?
Yes, several languages and tools can be used for web scraping, with Python (using libraries like BeautifulSoup and Scrapy) being one of the most popular alternatives.
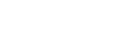
Jan 29, 2025
Was this helpful?